IVR application demo
Make a call to a fictitious logistics company using WebRTC or from your phone, and watch the application code unfold as it handles your call:
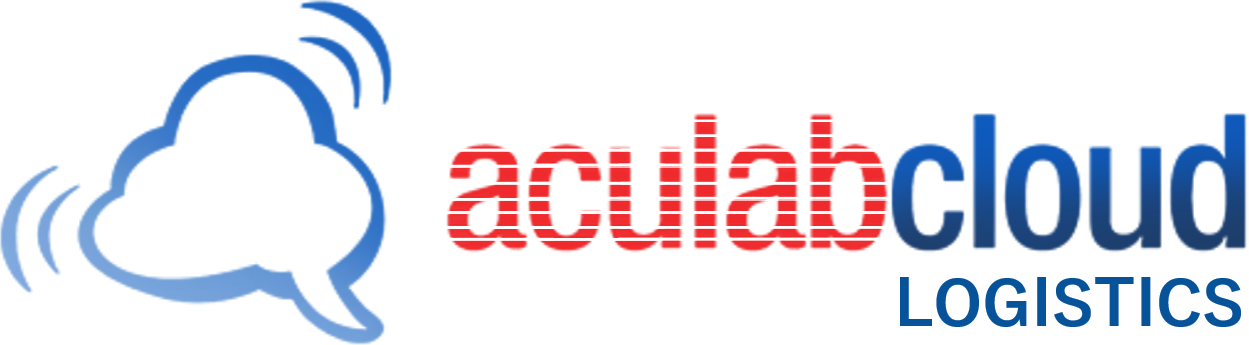
-
-
Python REST API
Create telephony applications quickly and easily using Aculab Cloud's REST API. Using our simple JSON language wrapper, you'll be able to call Cloud Actions such as Play() and Record(), helping you to write your interactive telephony application.
IVR applications can be created using the RunMenu() Action, allowing you to ask questions (using wav files or TTS), get answers from the telephone keypad and drive your application flow depending on those answers.
Each of the links above above is a web page that our application uses when required. To see how it works, call in using WebRTC or from your phone, and watch the application code unfold as it handles your call.
-
list_of_actions = [] list_of_actions.append(Play(text_to_say='Hello and welcome to ' 'the Aculab Cloud Logistics ' 'IVR system.')) list_of_actions.append(Redirect(WebPage(url='after_intro'))) my_response = TelephonyResponse(list_of_actions) return my_response.get_json()
-
list_of_actions = [] list_of_actions.append(Play(text_to_say='Main menu.')) menu_options = [] menu_options.append(MenuOption('1', WebPage(url='opening_times'))) menu_options.append(MenuOption('2', WebPage(url='location_package'))) menu_options.append(MenuOption('3', WebPage(url='connect_agent'))) main_menu = RunMenu(menu_options) menu_text = ("Press 1 to hear our store location and opening times, " "press 2 to find the location of your package, " "or press 3 to talk to one of our highly experienced " "and friendly agents. At any time, just hit star to " "hear the options again.") main_menu.set_prompt(Play(text_to_say=menu_text)) list_of_actions.append(main_menu) my_response = TelephonyResponse(list_of_actions) return my_response.get_json()
-
list_of_actions = [] list_of_actions.append(Play(text_to_say="Aculab Cloud Logistics " "are located at 2 Bramley Road, " "Milton Keynes, MK1 1PT, United " "Kingdom. Our working hours are " "Monday to Friday, " "9 a.m. to 5 p.m.")) list_of_actions.append(Redirect(WebPage(url='after_intro'))) my_response = TelephonyResponse(list_of_actions) return my_response.get_json()
-
list_of_actions = [] my_number = GetNumber(WebPage(url='location_package_process')) my_number.set_prompt(Play(text_to_say="Please enter your four " "digit package number to " "locate. For demonstration " "purposes, you can enter any " "number you like.")) my_number.set_digit_count(4) list_of_actions.append(my_number) my_response = TelephonyResponse(list_of_actions) return my_response.get_json()
-
my_request = TelephonyRequest(request) action_result = my_request.get_action_result() get_number_result = action_result.get("result") entered_number = get_number_result.get("entered_number") msg = ("Package " + ','.join(entered_number) + " was signed for " "by Allan yesterday at 3:30 p.m.") list_of_actions = [] list_of_actions.append(Play(text_to_say=msg)) list_of_actions.append(Redirect(WebPage(url='after_intro'))) my_response = TelephonyResponse(list_of_actions) return my_response.get_json()
-
list_of_actions = [] my_connect = Connect() my_connect.set_destinations(['sip:agent_ivr@sip-1-2-0.aculab.com']) my_connect.set_hold_media(Play(text_to_say="All of our operators are " "currently busy. Your call " "is important to us. Please " "hold the line, and an agent " "will answer shortly.")) list_of_actions.append(my_connect) list_of_actions.append(Redirect(WebPage(url='after_intro'))) my_response = TelephonyResponse(list_of_actions) return my_response.get_json()
-
list_of_actions = [] list_of_actions.append(Play(text_to_say="Hello, my name is Joey from " "Aculab Cloud Logistics. " "It's quite possible to connect " "your inbound application to " "another person using conferencing " "or using an outbound telephone " "call.")) list_of_actions.append(Play(text_to_say="I hope I've been able to help you " "with your inquiry. Thank you for " "using Aculab Cloud Logistics.")) my_response = TelephonyResponse(list_of_actions) return my_response.get_json()
-
-
-
C# REST API
Create telephony applications quickly and easily using Aculab Cloud's REST API. Using our simple JSON language wrapper, you'll be able to call Cloud Actions such as Play() and Record(), helping you to write your interactive telephony application.
IVR applications can be created using the RunMenu() Action, allowing you to ask questions (using wav files or TTS), get answers from the telephone keypad and drive your application flow depending on those answers.
Each of the links above above is a web page that our application uses when required. To see how it works, call in using WebRTC or from your phone, and watch the application code unfold as it handles your call.
-
List<TelephonyAction> actions = new List<TelephonyAction>(); Play playAction = Play.SayText("Hello and welcome to the " + "Aculab Cloud Logistics IVR system."); actions.Add(playAction); actions.Add(new Redirect(new WebPageRequest("after_intro"))); TelephonyResponse ourResponse = new TelephonyResponse(actions); ourResponse.ToHttpResponse(Response);
-
List<TelephonyAction> actions = new List<TelephonyAction>(); Play playAction = Play.SayText("Main menu."); actions.Add(playAction); List<MenuOption> options = new List<MenuOption> { new MenuOption('1', new WebPageRequest("opening_times")), new MenuOption('2', new WebPageRequest("location_package")), new MenuOption('3', new WebPageRequest("connect_agent")), }; RunMenu menu = new RunMenu(options); menu.Prompt = Play.SayText("Press 1 to hear our store " + "location and opening times, press 2 to find the " + "location of your package, or press 3 to talk to one " + "of our highly experienced and friendly agents. At " + "any time, just hit star to hear the options again."); actions.Add(menu); TelephonyResponse ourResponse = new TelephonyResponse(actions); ourResponse.ToHttpResponse(Response);
-
List<TelephonyAction> actions = new List<TelephonyAction>(); Play playAction = Play.SayText("Aculab Cloud Logistics " + "are located at 2 Bramley Road, Milton Keynes, " + "MK1 1PT, United Kingdom. Our working hours are " + "Monday to Friday, 9 a.m. to 5 p.m."); actions.Add(playAction); actions.Add(new Redirect(new WebPageRequest("after_intro"))); TelephonyResponse ourResponse = new TelephonyResponse(actions); ourResponse.ToHttpResponse(Response);
-
List<TelephonyAction> actions = new List<TelephonyAction>(); Play prompt = Play.SayText("Please enter your four digit " + "package number to locate. For demonstration purposes, " + "you can enter any number you like."); WebPageRequest nextPage = new WebPageRequest("location_package_process"); GetNumber getNumber = new GetNumber(nextPage, prompt, 4); actions.Add(getNumber); TelephonyResponse ourResponse = new TelephonyResponse(actions); ourResponse.ToHttpResponse(Response);
-
TelephonyRequest ourRequest = new TelephonyRequest(Request); InstanceInfo instanceInfo = ourRequest.InstanceInfo; GetNumberResult result = (GetNumberResult)instanceInfo.ActionResult; String enteredNumber = result.EnteredNumber; List<TelephonyAction> actions = new List<TelephonyAction>(); Play playAction = Play.SayText("Package " + String.Join(",", enteredNumber.ToCharArray()) + " was signed for by Allan yesterday at 3:30 p.m."); actions.Add(playAction); actions.Add(new Redirect(new WebPageRequest("after_intro"))); TelephonyResponse ourResponse = new TelephonyResponse(actions); ourResponse.ToHttpResponse(Response);
-
List<TelephonyAction> actions = new List<TelephonyAction>(); Connect connect = new Connect("sip:agent_ivr@sip-1-2-0.aculab.com"); connect.HoldMedia = Play.SayText("All of our operators are " + "currently busy. Your call is important to us. Please " + "hold the line, and an agent will answer shortly."); actions.Add(connect); actions.Add(new Redirect(new WebPageRequest("after_intro"))); TelephonyResponse ourResponse = new TelephonyResponse(actions); ourResponse.ToHttpResponse(Response);
-
List<TelephonyAction> actions = new List<TelephonyAction>(); Play playAction = Play.SayText("Hello, my name is Joey " + "from Aculab Cloud Logistics. It's quite possible to " + "connect your inbound application to another person " + "using conferencing or using an outbound telephone call."); actions.Add(playAction); playAction = Play.SayText("I hope I've been able to help " + "you with your inquiry. Thank you for using Aculab " + "Cloud Logistics."); actions.Add(playAction); TelephonyResponse ourResponse = new TelephonyResponse(actions); ourResponse.ToHttpResponse(Response);
-
-
-
Java REST API
Create telephony applications quickly and easily using Aculab Cloud's REST API. Using our simple JSON language wrapper, you'll be able to call Cloud Actions such as Play() and Record(), helping you to write your interactive telephony application.
IVR applications can be created using the RunMenu() Action, allowing you to ask questions (using wav files or TTS), get answers from the telephone keypad and drive your application flow depending on those answers.
Each of the links above above is a web page that our application uses when required. To see how it works, call in using WebRTC or from your phone, and watch the application code unfold as it handles your call.
-
List<TelephonyAction> actions = new ArrayList<TelephonyAction>(); actions.add(Play.sayText("Hello and welcome to " + "the Aculab Cloud Logistics " + "IVR system.")); actions.add(new Redirect(new WebPageRequest("after_intro"))); TelephonyResponse ourResponse = new TelephonyResponse(actions); ourResponse.setHttpServletResponse(response);
-
List<TelephonyAction> actions = new ArrayList<TelephonyAction>(); actions.add(Play.sayText("Main menu.")); Play prompt = Play.sayText("Press 1 to hear our store location and " + "opening times, press 2 to find the location of your package, " + "or press 3 to talk to one of our highly experienced and friendly " + "agents. At any time, just hit star to hear the options again."); List<MenuOption> options = new ArrayList<MenuOption>(); options.add(new MenuOption('1', new WebPageRequest("opening_times"))); options.add(new MenuOption('2', new WebPageRequest("location_package"))); options.add(new MenuOption('3', new WebPageRequest("connect_agent"))); RunMenu runMenuAction = new RunMenu(options); runMenuAction.setPrompt(prompt); actions.add(runMenuAction); TelephonyResponse ourResponse = new TelephonyResponse(actions); ourResponse.setHttpServletResponse(response);
-
List<TelephonyAction> actions = new ArrayList<TelephonyAction>(); actions.add(Play.sayText("Aculab Cloud Logistics " + "are located at 2 Bramley Road, " + "Milton Keynes, MK1 1PT, United " + "Kingdom. Our working hours are " + "Monday to Friday, " + "9 a.m. to 5 p.m.")); actions.add(new Redirect(new WebPageRequest("after_intro"))); TelephonyResponse ourResponse = new TelephonyResponse(actions); ourResponse.setHttpServletResponse(response);
-
List<TelephonyAction> actions = new ArrayList<TelephonyAction>(); Play prompt = Play.sayText("Please enter your four digit package " + "number to locate. For demonstration purposes, you can enter " + "any number you like."); WebPageRequest nextPage = new WebPageRequest("location_package_process"); actions.add(new GetNumber(nextPage, prompt, 4)); TelephonyResponse ourResponse = new TelephonyResponse(actions); ourResponse.setHttpServletResponse(response);
-
TelephonyRequest ourRequest = new TelephonyRequest(request); InstanceInfo instanceInfo = ourRequest.getInstanceInfo(); GetNumberResult numberResult = (GetNumberResult)instanceInfo.getActionResult(); String enteredNumber = numberResult.getEnteredNumber(); String msg = "Package " + StringUtils.join(enteredNumber.toCharArray(), ',') + " was signed for by Allan yesterday at 3:30 p.m."; List<TelephonyAction> actions = new ArrayList<TelephonyAction>(); actions.add(Play.sayText(msg)); actions.add(new Redirect(new WebPageRequest("after_intro"))); TelephonyResponse ourResponse = new TelephonyResponse(actions); ourResponse.setHttpServletResponse(response);
-
List<TelephonyAction> actions = new ArrayList<TelephonyAction>(); Connect connectAction = new Connect("sip:agent_ivr@sip-1-2-0.aculab.com"); connectAction.setHoldMedia(Play.sayText("All of our operators are " + "currently busy. Your call is important to us. Please hold the " + "line, and an agent will answer shortly.")); actions.add(connectAction); actions.add(new Redirect(new WebPageRequest("after_intro"))); TelephonyResponse ourResponse = new TelephonyResponse(actions); ourResponse.setHttpServletResponse(response);
-
List<TelephonyAction> actions = new ArrayList<TelephonyAction>(); actions.add(Play.sayText("Hello, my name is Joey from Aculab Cloud " + "Logistics. It's quite possible to connect your inbound " + "application to another person using conferencing or using an " + "outbound telephone call.")); actions.add(Play.sayText("I hope I've been able to help you with your " + "inquiry. Thank you for using Aculab Cloud Logistics.")); TelephonyResponse ourResponse = new TelephonyResponse(actions); ourResponse.setHttpServletResponse(response);
-
-
PHP REST API
Create telephony applications quickly and easily using Aculab Cloud's REST API. Using our simple JSON language wrapper, you'll be able to call Cloud Actions such as Play() and Record(), helping you to write your interactive telephony application.
IVR applications can be created using the RunMenu() Action, allowing you to ask questions (using wav files or TTS), get answers from the telephone keypad and drive your application flow depending on those answers.
Each of the links above above is a web page that our application uses when required. To see how it works, call in using WebRTC or from your phone, and watch the application code unfold as it handles your call.
-
$my_response = new Response(); $my_response->addAction(Play::sayText('Hello and welcome ' . 'to the Aculab Cloud Logistics IVR system.')); $my_response->addAction(new Redirect('after_intro')); print $my_response;
-
$my_response = new Response(); $my_response->addAction(Play::sayText('Main menu.')); $menu_text = Play::sayText("Press 1 to hear our store " . "location and opening times, press 2 to find the location " . "of your package, or press 3 to talk to one of our highly " . "experienced and friendly agents. At any time, just hit " . "star to hear the options again."); $main_menu = new RunMenu(); $main_menu->setPrompt($menu_text); $main_menu->addMenuOption('1', 'opening_times'); $main_menu->addMenuOption('2', 'location_package'); $main_menu->addMenuOption('3', 'connect_agent'); $my_response->addAction($main_menu); print $my_response;
-
$my_response = new Response(); $my_response->addAction(Play::sayText('Aculab Cloud Logistics ' . 'are located at 2 Bramley Road, Milton Keynes, MK1 1PT, ' . 'United Kingdom. Our working hours are Monday to Friday, ' . '9 a.m. to 5 p.m.')); $my_response->addAction(new Redirect('after_intro')); print $my_response;
-
$my_response = new Response(); $get_number = new GetNumber('location_package_process'); $get_number->setPrompt(Play::sayText('Please enter your four ' . 'digit package number to locate. For demonstration purposes, ' . 'you can enter any number you like.')); $get_number->setDigitCount(4); $my_response->addAction($get_number); print $my_response;
-
$instance_info = InstanceInfo::getInstanceInfo(); $entered_number = $instance_info->getActionResult()->getEnteredNumber(); $msg = 'Package ' . implode(',', str_split($entered_number)) . ' was signed for by Allan yesterday at 3:30 p.m.'; $my_response = new Response(); $my_response->addAction(Play::sayText($msg)); $my_response->addAction(new Redirect('after_intro')); print $my_response;
-
$my_response = new Response(); $connect = new Connect(); $connect->addDestination('sip:agent_ivr@sip-1-2-0.aculab.com'); $connect->setHoldMedia(Play::sayText('All of our operators are ' . 'currently busy. Your call is important to us. Please hold the ' . 'line, and an agent will answer shortly.')); $my_response->addAction($connect); $my_response->addAction(new Redirect('after_intro')); print $my_response;
-
$my_response = new Response(); $my_response->addAction(Play::sayText("Hello, my name is Joey from " . "Aculab Cloud Logistics. It's quite possible to connect your inbound " . "application to another person using conferencing or using an outbound " . "telephone call.")); $my_response->addAction(Play::sayText("I hope I've been able to help you " . "with your inquiry. Thank you for using Aculab Cloud Logistics.")); print $my_response;
-
-
-
Python UAS application
Create telephony applications quickly and easily using Aculab Cloud's UAS system. Write your programs in Python and import them into our User Application Server (UAS) to enable telephony functionality. We offer a high level wrapper to help write your interactive telephone application.
IVR applications can be created using the invoke_menu() functions, allowing you to set the prompt message (in either text or recorded wave file) and valid key presses. The function returns with the validated keypad character pressed, allowing you to then call another menu function.
Each of the links above above are sub-routines that our application uses when required. To see how it works, call in using WebRTC or from your phone, and watch the application code unfold as it handles your call.
-
def main(channel, application_instance_id, file_man, my_log, application_parameters): tts = "Hello and welcome to the Aculab Cloud Logistics IVR system." channel.FilePlayer.say (tts, barge_in = True) after_intro(channel) return 0;
-
def after_intro(channel): tts = "Main menu." channel.FilePlayer.say (tts) tts = "Press 1 to hear our store location and opening times, press 2 " \ "to find the location of your package, or press 3 to talk to one of our " \ "highly experienced and friendly agents. At any time, just hit star to " \ "hear the options again." channel_helper = HighLevelCallChannel (channel, my_log) digit = channel_helper.invoke_menu (PlayableMedia (text_to_say = tts), "123") if digit == '1': opening_times(channel) elif digit == '2': location_package(channel) elif digit == '3': connect_agent(channel)
-
def opening_times(channel): tts = "Aculab Cloud Logistics are located at 2 Bramley Road, " \ "Milton Keynes, MK1 1PT, United Kingdom. Our working hours are Monday " \ "to Friday, 9 a.m. to 5 p.m." channel.FilePlayer.say (tts)
-
def location_package(channel): channel_helper = HighLevelCallChannel (channel, my_log); tts = "Please enter your four digit package number to locate. For " \ "demonstration purposes, you can enter any number you like." digits = channel_helper.capture_validated_number (PlayableMedia(text_to_say = tts), dtmf_count = 4) ivr_demo_location_package_process (channel, digits);
-
def ivr_demo_location_package_process(channel, digits): if digits: tts = "Package " + ','.join (digits) + " was signed for " \ "by Allan yesterday at 3:30 p.m." channel.FilePlayer.say (tts)
-
def connect_agent(channel): tts = "All of our operators are currently busy. Your call is " \ important to us. Please hold the line and an agent will answer shortly." channel.FilePlayer.say(tts) channel_helper = HighLevelCallChannel (channel, my_log) channel_helper.call_inbound_service_and_connect ( channel.ExtraChannel[0], call_to = 'sip:agent_ivr@sip-1-2-0.aculab.com')
-
def main(channel, application_instance_id, file_man, my_log, application_parameters): tts = "Hello, my name is Joey from Aculab Cloud Logistics. " \ "It's quite possible to connect your inbound application to " \ "another person using conferencing or using an outbound telephone " \ "call. I hope I've been able to help you with your inquiry." \ "Thank you for using Aculab Cloud Logistics." channel.FilePlayer.say (tts)
-
-
-
C# UAS application
Create telephony applications quickly and easily using Aculab Cloud's UAS system. Write your programs in C# and import them into our User Application Server (UAS) to enable telephony functionality. We offer a high level wrapper to help write your interactive telephone application.
IVR applications can be created using the InvokeMenu() functions, allowing you to set the prompt message (in either text or recorded wave file) and valid key presses. The function returns with the validated keypad character pressed, allowing you to then call another menu function.
Each of the links above above are sub-routines that our application uses when required. To see how it works, call in using WebRTC or from your phone, and watch the application code unfold as it handles your call.
-
public override int Run(UASCallChannel channel, string applicationParameters) { String tts = "Hello and welcome to the the Aculab Cloud Logistics IVR."; channel.FilePlayer.Say(tts); after_intro(channel); return 0; }
-
public void after_intro(UASCallChannel channel) { CallChannelHelper channelHelper = new CallChannelHelper(this, channel); String tts = "Press 1 to hear our store location and opening times, " + "press 2 to find the location of your package, or press 3 to talk to one " + "of our highly experienced and friendly agents. At any time, just hit star " + "to hear the options again."; PlayableMedia menu = PlayableMedia.TextToSay(tts); char selection = channelHelper.InvokeMenu(menu, "123"); if (selection == '1') opening_times(channel); else if (selection == '2') location_package(channel); else if (selection == '3') connect_agent(channel); }
-
public void after_intro(UASCallChannel channel) { String tts = "Aculab Cloud Logistics are located at 2 Bramley Road, " + "Milton Keynes, MK1 1PT, United Kingdom. Our working hours are Monday " + "to Friday, 9 a.m. to 5 p.m."; channel.FilePlayer.Say(tts); }
-
public void location_package(UASCallChannel channel) { CallChannelHelper channelHelper = new CallChannelHelper(this, channel); String tts = "Please enter your four digit package number to locate. " + "For demonstration purposes, you can enter any number you like."; PlayableMedia prompt = PlayableMedia.TextToSay(tts); String number = channelHelper.CaptureNumber(prompt, 4); if (number.Length == 4) location_package_process(number); }
-
public void location_package_process(UASCallChannel channel) { if (String.IsNullOrEmpty(number)) return; String package = String.Join(",", number.ToCharArray()); String tts = "Package " + package + " was signed for by Allan yesterday at 3:30 p.m."; channel.FilePlayer.Say(tts); }
-
public void connect_agent(UASCallChannel channel) { CallChannelHelper channelHelper = new CallChannelHelper(this, channel); String tts = "All of our operators are currently busy. Your call is " + "important to us. Please hold the line and an agent will answer shortly."; channel.FilePlayer.Say(tts); CallTarget callTarget = CallTarget.SipAddressToCall("sip:agent_ivr@sip-1-2-0.aculab.com", null); channelHelper.CallAndConnect(callTarget, channel.ExtraChannels[0], 120); WaitForAnyCallIdle(new UASCallChannel[] {{ channel, channel.ExtraChannels[0] }}, 600); }
-
public override int Run(UASCallChannel channel, string applicationParameters) { String tts = "Hello, my name is Joey from Aculab Cloud Logistics. " + "It's quite possible to connect your inbound application to another person " + "using conferencing or using an outbound telephone call. I hope I've been able " + "to help you with your inquiry. Thank you for using Aculab Cloud Logistics."; channel.FilePlayer.Say(tts); return 0; }
-
-