Starting An Outbound Service
You start your outbound application by invoking your Outbound Service on Aculab Cloud that refers to your application by name. You can do this manually from the Outbound Services page or you can call a web service on Aculab Cloud to start it.
Aculab Cloud makes an outbound call and when it is answered it requests your application's First Page.
If far-end classification is enabled, when the call is answered Aculab Cloud will attempt to classify the far-end. If answering machine be detected it wait for the machine's prompt message to be played before requesting your application's First Page.
Note: An Outbound Service is configured either to call a Telephone Number/SIP address or a conference. If configured incorrectly, the outbound call will fail.
Starting an Outbound Service from the Outbound Services page
Press the gearwheel icon next to your outbound service on the Outbound Services page. You should see a form like this one;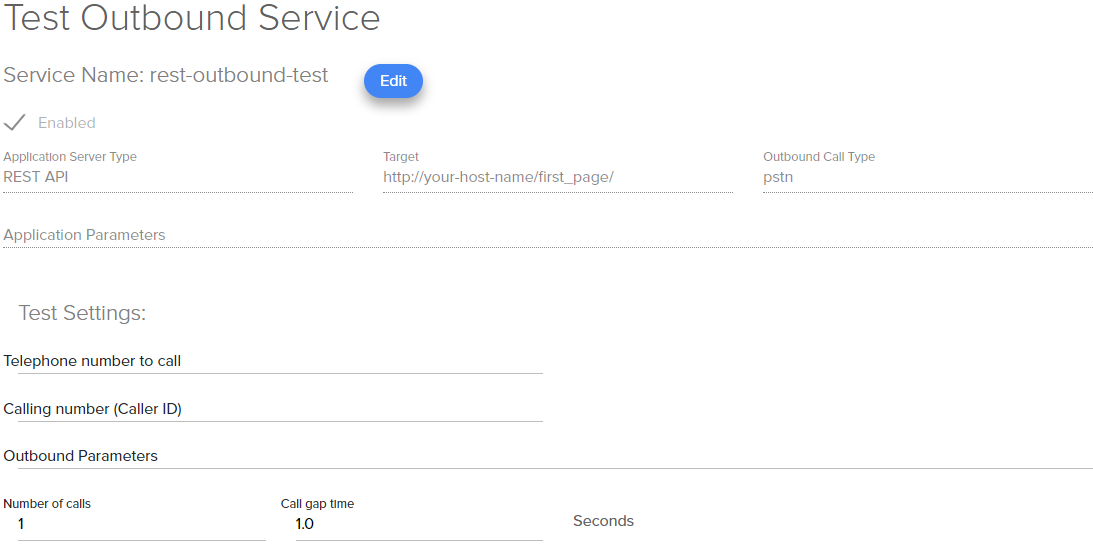
Starting an Outbound Service using the service_start Web Service
The outbound parameters argument for service_start, when used to start a REST API service, requires a JSON format string that specifies the destination of the call. This can either be a single JSON object containing the following parameters or an array of JSON objects each containing the following parameters. If an array is provided, then a new instance of the service is started for each object provided:
Property | Required/Optional | Description |
---|---|---|
to | required | A string containing the destination for the outbound call. This can be a phone number, SIP address or conference room name. |
from | required only if to is a telephone number |
A string containing the origin telephone number (or SIP address) from which the call is being made.
If to is a telephone number, then this needs to be a validated caller id (see Caller IDs).
|
token | optional | A string containing a user-defined token that will be passed transparently to the Web App in the
token property of the instance_info argument to the page request.
|
outbound parameters | optional | A string containing user-defined data that will be passed transparently to the Web App in the this_call.outbound_parameters
property of the instance_info argument to the page request.
|
Http authentication username and password:
The authentication username and password contain details of the service to call and the account on which it is configured. The username contains three elements delimited by '/':
cloudId / account username / name of the outbound service
The password should be the outbound service password specified for the selected outbound service.
e.g.Username: 1-2-0/user@example.com/MyOutboundService
Password: MyOutboundServicePassword
High-level Language Wrappers
The service_start web service can be called using one of our high-level language wrappers:
-
Examples:
-
Using the service_start web service to call a telephone number:
https://ws.aculabcloud.net/service_start?outbound_parameters= { "to" : "441908283800", "from" : "441908283801", "token" : "my token info", "outbound_parameters" : "some user data" }
with:
Username: cloudId/accountName/outboundServiceName (e.g. 1-2-0/user@example.com/MyOutboundService) Password: outboundServicePassword
-
Using the service_start web service to call a conference:
https://ws.aculabcloud.net/service_start?outbound_parameters= { "to" : "myConference", "token" : "my token info", "outbound_parameters" : "some user data" }
with:
Username: cloudId/accountName/outboundServiceName (e.g. 1-2-0/user@example.com/MyOutboundService) Password: outboundServicePassword
-
Using the service_start web service to start multiple instances of a service each of which will call one of the specified destinations:
https://ws.aculabcloud.net/service_start?outbound_parameters= [ { "to" : "441908283800", "from" : "441908283801", "token" : "token1", "outbound_parameters" : "some user data" }, { "to" : "sip:bob@acompany.com", "token" : "token2" }, { "to" : "sip:bill@anotherCompany.com", "from" : "sip:bob@acompany.com", "token" : "token3" } ]
with:
Username: cloudId/accountName/outboundServiceName (e.g. 1-2-0/user@example.com/MyOutboundService) Password: outboundServicePassword
-
-
class OutboundRESTAPIService
Constructors:
OutboundRESTAPIService(WebServiceWrappers.PlatformAccount account, String serviceName, String servicePassword); OutboundRESTAPIService(String connection, String username, String serviceName, String servicePassword);
Members:
List<ServiceInvocation> Start(OutboundCall call); List<ServiceInvocation> Start(List<OutboundCall> calls); List<ServiceInvocation> UpdateStatus(out bool finished); bool Finished;
class OutboundCall
Constructors:
OutboundCall(String to); OutboundCall(String to, String from);
Members:
String Token; String OutboundParameters;
class ServiceInvocation
Members:
String ApplicationInstanceId; bool Finished; ServiceStatus Status; String ReturnText; int ReturnCode; DateTime ApplicationStatusEta;
Examples:
-
Use the OutboundRESTAPIService class to call a telephone number:
// Create the service object OutboundRESTAPIService myService = new OutboundRESTAPIService("1-2-0", "bob@acompany.com", "myOutboundService", "myOutboundServicePassword"); // Create the target OutboundCall call = new OutboundCall("441908273800", "441908273801"); call.Token = "my token info"; call.OutboundParameters = "some user data"; // Start the service List<ServiceInvocation> invocations = myService.Start(call);
-
Use the OutboundRESTAPIService class to call a conference:
// Create the service object OutboundRESTAPIService myService = new OutboundRESTAPIService("1-2-0", "bob@acompany.com", "myCallAConferenceService", "myCallAConferenceServicePassword"); // Create the target OutboundCall call = new OutboundCall("myConference"); call.Token = "my token info"; call.OutboundParameters = "some user data"; // Start the service List<ServiceInvocation> invocations = myService.Start(call);
-
Use the OutboundRESTAPIService class to start multiple instances of a service each of which will call a different destination. Wait for all the service instances to complete.:
// Create the service object OutboundRESTAPIService myService = new OutboundRESTAPIService("1-2-0", "bob@acompany.com", "myOutboundService", "myOutboundServicePassword"); // Create multiple targets List<OutboundCall> calls = new List<OutboundCall>(3); calls.Add(new OutboundCall("441908273800", "441908273801")); calls[0].Token = "my token info 1"; calls[0].OutboundParameters = "some user data"; calls.Add(new OutboundCall("sip:bob@acompany.com")); calls[1].Token = "my token info 2"; calls.Add(new OutboundCall("sip:bill@anothercompany.com", "sip:bob@acompany.com")); calls[2].Token = "my token info 3"; // Start the services List<ServiceInvocation> invocations = myService.Start(call2); // Wait until all instances of this service run have completed. bool finished; do { invocations = myService.UpdateStatus(out finished); Thread.Sleep(2000); } while (!finished);
-
-
Class OutboundRESTAPIService
Constructors:
New(account As WebServiceWrappers.PlatformAccount, serviceName As String, servicePassword As String) New(connection As String, username As String, serviceName As String, servicePassword As String)
Members:
Start(call As OutboundCall) As List(Of ServiceInvocation) Start(calls As List(Of OutboundCall)) As List(Of ServiceInvocation) UpdateStatus(ByRef finished As Boolean) As List(Of ServiceInvocation) Finished As Boolean
Class OutboundCall
Constructors:
New(to As String) New(to As String, from As String)
Members:
Token As String OutboundParameters As String
Class ServiceInvocation
Members:
Finished As Boolean Status As WebServiceWrappers.ServiceStatus ApplicationInstanceId As String ReturnText As String ReturnCode As Integer ApplicationStatusEta As DateTime
Examples:
-
Use the service_start web service to call a telephone number:
' Create the service object Dim myService As OutboundRESTAPIService = New OutboundRESTAPIService("1-2-0", "bob@acompany.com", "myOutboundService", "myOutboundServicePassword") ' Create the target Dim call As OutboundCall = New OutboundCall("441908273800", "441908273801") call.Token = "my token info" call.OutboundParameters = "some user data" ' Start the service Dim invocations As List(Of ServiceInvocation) = myService.Start(call)
-
Use the service_start web service to call a conference:
' Create the service object Dim myService As OutboundRESTAPIService = New OutboundRESTAPIService("1-2-0", "bob@acompany.com", "myCallAConferenceService", "myCallAConferenceServicePassword") ' Create the target Dim call As OutboundCall = New OutboundCall("myConference") call.Token = "my token info" call.OutboundParameters = "some user data" ' Start the service Dim invocations As List(Of ServiceInvocation) = myService.Start(call)
-
Use the service_start web service to start multiple instances of a service each of which will call a different destination. Wait for all the service instances to complete:
' Create the service object Dim myService As OutboundRESTAPIService = New OutboundRESTAPIService("1-2-0", "bob@acompany.com", "myOutboundService", "myOutboundServicePassword") ' Create multiple targets Dim calls List(Of OutboundCall) = New List(Of OutboundCall)(3) calls.Add(New OutboundCall("441908273800", "441908273801")) calls(0).Token = "my token info 1" calls(0).OutboundParameters = "some user data" calls.Add(New OutboundCall("sip:bob@acompany.com")) calls(1).Token = "my token info 2" calls.Add(New OutboundCall("sip:bill@anothercompany.com", "sip:bob@acompany.com")) calls(2).Token = "my token info 3" ' Start the services Dim invocations As List(Of ServiceInvocation) = myService.Start(calls) ' Wait until all instances of this service run have completed. Dim finished As Boolean Do invocations = myService.UpdateStatus(finished) Thread.Sleep(2000) Loop while Not finished
-
-
class OutboundRESTAPIService
Constructors:
OutboundRESTAPIService(String connection, String cloudUsername, String serviceName, String servicePassword)
Members:
List<ServiceInvocation> start(OutboundCall call); List<ServiceInvocation> start(List<OutboundCall> calls); List<ServiceInvocation> updateStatus();
class OutboundCall
Constructors:
OutboundCall(String to); OutboundCall(String to, String from);
Members:
void setToken(String token); void setOutboundParameters(String outboundParameters);
class ServiceInvocation
Members:
boolean isFinished(); ServiceStatus getStatus(); String getApplicationInstanceId(); String getReturnText();
Examples:
-
Use the service_start web service to call a telephone number:
// Create the service object OutboundRESTAPIService myService = new OutboundRESTAPIService("1-2-0", "bob@acompany.com", "myOutboundService", "myOutboundServicePassword"); // Create the target OutboundCall call = new OutboundCall("441908273800", "441908273801"); call.setToken("my token info"); call.setOutboundParameters("some user data"); // Start the service List<ServiceInvocation> invocations = myService.start(call);
-
Use the service_start web service to call a conference:
// Create the service object OutboundRESTAPIService myService = new OutboundRESTAPIService("1-2-0", "bob@acompany.com", "myCallAConferenceService", "myOutboundServicePassword"); // Create the target OutboundCall call = new OutboundCall("myConference"); call.setToken("my token info"); call.setOutboundParameters("some user data"); // Start the service List<ServiceInvocation> invocations = myService.start(call);
-
Use the service_start web service to start multiple instances of a service each of which will call a differnet destination. Wait for all the service instances to complete:
// Create the service object OutboundRESTAPIService myService = new OutboundRESTAPIService("1-2-0", "bob@acompany.com", "myCallAConferenceService", "myOutboundServicePassword"); // Create multiple targets List<OutboundCall> calls = new ArrayList<OutboundCall>(3); calls.add(new OutboundCall("441908273800", "441908273801")); calls[0].setToken("my token info 1"); calls[0].setOutboundParameters("some user data"); calls.add(new OutboundCall("sip:bob@acompany.com")); calls[1].setToken("my token info 2"); calls.add(new OutboundCall("sip:bill@anothercompany.com", "sip:bob@acompany.com")); calls[2].setToken("my token info 3"); // Start the services List<ServiceInvocation> invocations = myService.start(call2); // Wait until all instances of this service run have completed. do { invocations = myService.updateStatus(); Thread.sleep(2000); } while (!myService.isFinished());
-
-
Starting an outbound service.
The python web_services module exposes a function called invoke_outbound_service and a class called OutboundCalls.invoke_outbound_service(target, username, service_name, service_password, outbound_calls):
Arguments:
Parameter Name Description target The id of the cloud you want to connect to, e.g, 1-2-0. username Your username on the target, e.g, my@email.address. service_name The name of the outbound service you want to invoke. service_password Your outbound service password as set in the outbound services page. outbound_calls This is an instance of a class of type OutboundCalls class OutboundCalls
Constructors:
OutboundCalls(call_to, call_from=None, token=None, outbound_parameters=None)
Members:
def add_call(self, call_to, call_from=None, token=None, outbound_parameters=None):
Parameter Name Description call_to The call destination, a telephone number or SIP address. call_from The call origin. If calling a telephone number this might have to be a validated number. token A string that will be passed to your application as part of the instance info object. outbound_parameters Additional arguments that you want passed to the outbound application that will be triggered by the outbound service. def get_calls(self):
Examples:
-
Using the service_start web service to call a telephone number:
from web_services import invoke_outbound_service, OutboundCalls outbound_calls = OutboundCalls(call_to="441908273800", call_from="441908273801", token="my token info", outbound_parameters="some additional data") invoke_outbound_service(target='1-2-0', username="bob@acompany.com", service_name="myOutboundService", service_password="myOutboundServicePassword", outbound_calls=outbound_calls)
-
Using the service_start web service to call a conference:
from web_services import invoke_outbound_service, OutboundCalls outbound_calls = OutboundCalls(call_to="myConference", token="my token info", outbound_parameters="some additional data") # Please note that the outbound service must be configured as a conference destination invoke_outbound_service(target='1-2-0', username="bob@acompany.com", service_name="myOutboundConferenceService", service_password="myOutboundServicePassword", outbound_calls=outbound_calls)
-
Using the service_start web service to start multiple instances of a service each of which will call one of the specified destinations:
from web_services import invoke_outbound_service, OutboundCalls outbound_calls = OutboundCalls(call_to="441908273800", call_from="441908273801", token="my token info 1", outbound_parameters="some additional data") outbound_calls.add_call(call_to="sip:bob@acompany.com", token="my token info 2", outbound_parameters="some additional data") outbound_calls.add_call(call_to="sip:bill@anothercompany.com", call_from="sip:bob@acompany.com", token="my token info 3", outbound_parameters="some additional data") invoke_outbound_service(target='1-2-0', username="bob@acompany.com", service_name="myOutboundService", service_password="myOutboundServicePassword", outbound_calls=outbound_calls)
-
-
The OutboundService class
Class synopsis
class OutboundService extends WebServiceBase{ /* methods */ public __construct(string $target, string $user_name, string $service_name, string $service_password) public mixed startInstance(mixed $instanceConfigurations) public mixed getInstanceStatus(mixed $instanceIds) /* inherited methods */ public void setProxy(string $proxy_uri, boolean $request_fulluri) public void setErrorCallback(callable $callback) }
Description
Represents a configured outbound service.
The$target
of the constructor is a cloud identifier (such as '1-2-0')
startInstance()
takes either a singleOutboundInstanceConfiguration
or an array of them. Upon success it returns an array of strings, each string being an instance identifier that can be passed togetInstanceStatus()
. Upon failure, the return value is either an integer that is the HTTP response code or the valuefalse
getInstanceStatus()
takes either a single string or an array of strings. Each string being an instance identifier that came from a call tostartInstance()
. Upon success it returns an array ofOutboundInstanceStatus
objects keyed by the instance identifier strings. Upon failure, the return value is either an integer that is the HTTP response code or the valuefalse
setErrorCallback()
can be used to set a callback that is called when a HTTP error occurs. The callback is called with two parameters, the HTTP response code as an integer and the HTTP response body as a string. This is intended to aid debugging.The OutboundInstanceConfiguration class
Class synopsis
class OutboundInstanceConfiguration { /* methods */ public __construct(string $to, string $from = '') public void setToken(string $token) public void setOutboundParameters(string $outbound_parameters) }
Description
Represents the configuration used to start an outbound instance.The OutboundInstanceStatus class
Class synopsis
class OutboundInstanceStatus { /* methods */ public boolean isFinished() public string getStatus() public string getStatusDetail() public DateTime getApplicationStatusETA() public string getApplicationInstanceId() }
Description
Represents the status of an outbound instance.Examples:
-
Using the service_start web service to call a telephone number:
$service = new Aculab\TelephonyRestAPI\OutboundService('1-2-0', 'user@example.com', 'appname', 'pass'); $instconf = new Aculab\TelephonyRestAPI\OutboundInstanceConfiguration('441908283800', '441908283801'); $instconf->setToken('my token info'); $instconf->setOutboundParameters('some user data'); $ids = $service->startInstance($instconf);
-
Using the service_start web service to call a conference:
$service = new Aculab\TelephonyRestAPI\OutboundService('1-2-0', 'user@example.com', 'appname', 'pass'); $instconf = new Aculab\TelephonyRestAPI\OutboundInstanceConfiguration('myConference'); $instconf->setToken('my token info'); $instconf->setOutboundParameters('some user data'); $ids = $service->startInstance($instconf);
-
Using the service_start web service to start multiple instances of a service each of which will call one of the specified destinations:
$service = new Aculab\TelephonyRestAPI\OutboundService('1-2-0', 'user@example.com', 'appname', 'pass'); $inst1 = new Aculab\TelephonyRestAPI\OutboundInstanceConfiguration('441908283800', '441908283801'); $inst1->setToken('token1'); $inst1->setOutboundParameters('some user data'); $inst2 = new Aculab\TelephonyRestAPI\OutboundInstanceConfiguration('sip:bob@acompany.com'); $inst2->setToken('token2'); $inst3 = new Aculab\TelephonyRestAPI\OutboundInstanceConfiguration('sip:bill@anotherCompany.com', 'sip:bob@acompany.com'); $inst3->setToken('token3'); $instconfs = array($inst1, $inst2, $inst3); $ids = $service->startInstance($instconfs);
-