connect Action
Connects an existing inbound or outbound call (primary call) to a new call destination (secondary call). More than one possible destination can be specified, but only one secondary call ends up being connected. This is typically the first call to be answered.
language wrappers and examples
Note that web apps that use the connect
action require sufficient Extra Channels
to be set in the service configuration for the number of destinations specified.
The connect properties are:
Property | Required/Optional | Default | Description |
---|---|---|---|
destinations | required | An array of strings each specifying a destination, such as a telephone number, sip address, inbound service address or webRTC client Id. The first to answer is connected. The rest are hung up. An optional prefix may be supplied to declare a specific type of number/address, see Outbound Calls. | |
call from | optional | a number or address that identifies the primary call | A telephone number or sip address that identifies who is calling. If destinations contains one or more telephone numbers then you may need to supply a validated caller id here. See Caller IDs. |
hold media | optional | - | A play action. Media to play repeatedly to the primary call until the secondary call is connected or, if pass early media is true, early media is available. null signifies playing ringing to the call. |
pass early media | optional | false | true or false. Whether to pass early media from the secondary call to the primary call. Passing early media requires that only one destination is given, and that the secondary call does not have a first page specified. |
seconds answer timeout | optional | 30 | An integer. The time to wait for the secondary call to be answered. |
classify callee | optional | - | A classify callee object that determines whether and how to classify the far end of the secondary call. null signifies that classification is disabled. |
secondary call | optional | - | A secondary call object that specifies pages and properties that are related to the lifetime of the secondary call. |
translator | optional | - | A translator object that specifies an AI translator to be added to the connection. Both connected calls will hear the translator as well as each other. null signifies that a translator is not added to the connection. |
next page | optional | - | A web page request object that defines the web page to be requested once the connect has finished. If null or no page is specified or the secondary call fails to connect then the subsequent action in the action array will be executed. |
Returns
The result of a successfulconnect
action will be returned via the subsequent http request to the next page
in action result
as follows:Property | Availability | Description |
---|---|---|
seconds connected duration | always | A floating point value to one decimal place. The duration for which the two calls were connected. |
Remarks
The connect action makes outbound (secondary) calls to all specified destinations. As soon as one is answered either it is immediately connected to the primary call or, if a secondary callfirst page
is specified, the answered call's details are sent to it. Meanwile the other call attempts are dropped.If classification with
hang up on answering machine
is enabled, destinations that are classified as "answering_machine" will also be dropped and listed in the dropped calls
array sent to the secondary call final page
. Similarly, if classification with hang up on fax machine
is enabled, destinations that are classified as "fax_machine" will also be dropped.Setting the
first page
property allows the application to interact with the secondary call before it is connected as illustrated
in the following diagram: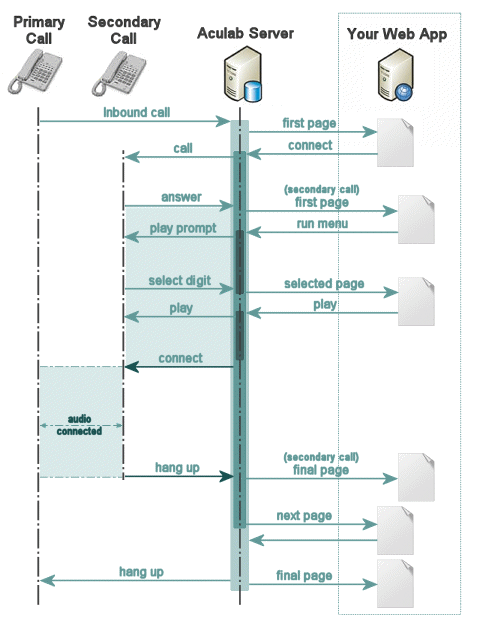
In this example a first page
is specified that responds with a run menu
action. The selected option's page then returns a further play action. Once this has completed the primary and secondary calls are connected.
When the secondary call goes idle its final page
is requested.
The this call
property in the request contains details of the secondary call.
Note: if the primary call hangs up, the secondary call is automatically hung up.
Subsequently the connect's next page
is requested. The action result
contains details of the connect. Note: If no next page
is defined then the application continues with the next action in the action array.
If interaction with any of the secondary call's pages fails then a request is made to the secondary call's error page
with a description of the issues.
-
Examples:
Make a call to a single destination, without classifying the callee, and connect it to the existing call:
"connect" : { "destinations" : [ "443069990123" ], "call_from" : "443069999876", "next_page" : { "url" : "connectNextPage" } }
Once the connect action has finished the duration for which the two calls were connected is specified in the request to the next page:
"action_result" : { "action" : "connect", "result" : { "seconds_connected_duration" : "47.3" } }
Connect one of two calls, classifying the callee. If it is an answering machine wait for the message to finish but hang up if it's a fax machine:
"connect" : { "destinations" : [ "443069990123", "443069990124" ], "call_from" : "443069999876", "hold_media" : { "play" : { "play_list" : [ { "file_to_play" : "welcome.wav" } ] } }, "seconds_answer_timeout" : 20, "secondary_call" : { "call_recording" : true, "minutes_max_call_duration" : 60 }, "classify_callee" : { "milliseconds_timeout" : 3000, "hang_up_on_answering_machine" : false, "hang_up_on_fax_machine" : true, "answering_machine_ready_to_record" : { "milliseconds_min_beep_duration" : 180, "milliseconds_post_beep_silence" : 1000, "milliseconds_no_beep_silence" : 4000, "seconds_max_message_duration" : 10 } }, "next_page" : { "url" : "connectNextPage" } }
Make two calls, requesting connectFirstPage when the first of the two calls is answered. Connect this call once all connectFirstPage's actions have run. Request connectFinalPage then connectNextPage once the connected call hangs up:
"connect" : { "destinations" : [ "443069990123", "443069990124" ], "call_from" : "443069999876", "hold_media" : { "play" : { "play_list" : [ { "file_to_play" : "welcome.wav" } ] } }, "seconds_answer_timeout" : 20, "secondary_call" : { "call_recording" : true, "minutes_max_call_duration" : 60, "first_page" : { "url" : "connectFirstPage" }, "final_page" : { "url" : "connectFinalPage" }, "error_page" : { "url" : "connectErrorPage" } }, "next_page" : { "url" : "connectNextPage" } }
Make a call, passing any early media from the secondary call to the primary call:
"connect" : { "destinations" : [ "443069990123" ], "call_from" : "443069999876", "pass_early_media" : true, "secondary_call" : { "call_recording" : true, "minutes_max_call_duration" : 60, "final_page" : { "url" : "connectFinalPage" }, "error_page" : { "url" : "connectErrorPage" } }, "next_page" : { "url" : "connectNextPage" } }
-
Make a call with a translator:
"connect" : { "destinations" : [ "443069990123" ], "call_from" : "443069999876", "translator" : { "primary" : { "speech_recognition_options" : { "language" : "en-GB", "speech_model_options" : { "model" : "latest_long" } }, "tts_voice" : "French France Female Polly Lea" }, "secondary" : { "speech_recognition_options" : { "language" : "fr-FR", "speech_model_options" : { "model" : "phone_call", "enhanced" : true } }, "tts_voice" : "English UK Female Polly Amy" }, "transcription_results_page" : { "url" : "transcriptionResultsPage" }, "id" : "1234" }, "next_page" : { "url" : "connectNextPage" } }
-
Connect Class
Namespace: Aculab.Cloud.RestAPIWrapper
Assembly: Aculab.Cloud.RestAPIWrapper.dllAn action to connect an existing inbound or outbound (primary) call to a new (secondary) call destination.
-
public class Connect : TelephonyAction { // Constructors public Connect(string destination); // Members public void AddDestination(string destination); public string CallFrom; public Play HoldMedia; public bool PassEarlyMedia; public int SecondsAnswerTimeout; public void EnableCalleeClassification(); public void EnableCalleeClassification(ClassifyCallee classifyCallee); public SecondaryCall SecondaryCall; public Translator Translator; public WebPageRequest NextPage; }
Examples:
-
Make a call to a single destination, without classifying the callee, and connect it to the existing call:
List<TelephonyAction> actions = new List<TelephonyAction>(); var connectAction = new Connect("443069990123") { CallFrom = "443069999876", NextPage = new WebPageRequest("ConnectNextPage.aspx") }; actions.Add(connectAction);
Once the connect action has finished the duration for which the two calls were connected is specified in the request to the next page:
// Unpack the request var telephonyRequest = new TelephonyRequest(Request); var connectResult = (ConnectResult)telephonyRequest.InstanceInfo.ActionResult; double connectedDuration = connectResult.SecondsConnectedDuration;
-
Connect one of two calls, classifying the callee. If it is an answering machine wait for the message to finish but hang up if it's a fax machine:
List<TelephonyAction> actions = new List<TelephonyAction>(); var connectAction = new Connect("443069990123") { CallFrom = "443069999876", HoldMedia = Play.PlayFile("welcome.wav"), SecondsAnswerTimeout = 20, SecondaryCall = new SecondaryCall(null, 60, true), NextPage = new WebPageRequest("ConnectNextPage.aspx") }; var classifyCallee = new ClassifyCallee(3000, false); classifyCallee.ConfigureAnsweringMachineReadyToRecord(new AnsweringMachineReadyToRecord(180, 1000, 4000, 10)); connectAction.EnableCalleeClassification(classifyCallee); connectAction.AddDestination("443069990124"); actions.Add(connectAction);
-
Make two calls, requesting connectFirstPage when the first of the two calls is answered. Connect this call once all connectFirstPage's actions have run. Request connectFinalPage then connectNextPage once the connected call hangs up:
List<TelephonyAction> actions = new List<TelephonyAction>(); var connectAction = new Connect("443069990123") { CallFrom = "443069999876", HoldMedia = Play.PlayFile("welcome.wav"), SecondsAnswerTimeout = 20, SecondaryCall = new SecondaryCall(new WebPageRequest("ConnectFirstPage.aspx"), 60, true) { FinalPage = new WebPageRequest("ConnectFinalPage.aspx"), ErrorPage = new WebPageRequest("ConnectErrorPage.aspx") } }; connectAction.AddDestination("443069990124"); actions.Add(connectAction);
-
Make a call, passing any early media from the secondary call to the primary call:
List<TelephonyAction> actions = new List<TelephonyAction>(); var connectAction = new Connect("443069990123") { CallFrom = "443069999876", PassEarlyMedia = true, SecondaryCall = new SecondaryCall() { CallRecording = true, MinutesMaxCallDuration = 60, FinalPage = new WebPageRequest("ConnectFinalPage.aspx"), ErrorPage = new WebPageRequest("ConnectErrorPage.aspx") }, NextPage = new WebPageRequest("ConnectNextPage.aspx") }; actions.Add(connectAction);
-
Make a call with a translator:
List<TelephonyAction> actions = new List<TelephonyAction>(); // How the primary call handles translation var primaryCallTranslationOptions = new CallTranslationOptions() { SpeechRecognitionOptions = new SpeechRecognitionOptions() { Language = "en-GB", SpeechModelOptions = new SpeechModelOptions(model : "latest_long") }, TTSVoice = "French France Female Polly Lea" }; // How the secondary call handles translation var secondaryCallTranslationOptions = new CallTranslationOptions() { SpeechRecognitionOptions = new SpeechRecognitionOptions() { Language = "fr-FR", SpeechModelOptions = new SpeechModelOptions(model : "phone_call", enhanced : true) }, TTSVoice = "English UK Female Polly Amy" }; var connectAction = new Connect("443069990123") { CallFrom = "443069999876", SecondaryCall = new SecondaryCall() { CallRecording = true, MinutesMaxCallDuration = 60, FinalPage = new WebPageRequest("ConnectFinalPage.aspx"), ErrorPage = new WebPageRequest("ConnectErrorPage.aspx") }, Translator = new Translator(primaryCallTranslationOptions, secondaryCallTranslationOptions) { TranscriptionResultsPage = new WebPageRequest("transcriptionResultsPage.aspx"), Id = "1234" }, NextPage = new WebPageRequest("ConnectNextPage.aspx") }; actions.Add(connectAction);
-
-
public class Connect : TelephonyAction { // Constructors public Connect(string destination); // Members public void AddDestination(string destination); public string CallFrom; public Play HoldMedia; public bool PassEarlyMedia; public int SecondsAnswerTimeout; public void EnableCalleeClassification(); public void EnableCalleeClassification(ClassifyCallee classifyCallee); public SecondaryCall SecondaryCall; public Translator Translator; public WebPageRequest NextPage; }
Examples:
-
Make a call to a single destination, without classifying the callee, and connect it to the existing call:
List<TelephonyAction> actions = new List<TelephonyAction>(); var connectAction = new Connect("443069990123") { CallFrom = "443069999876", NextPage = new WebPageRequest("ConnectNextPage.aspx") }; actions.Add(connectAction);
Once the connect action has finished the duration for which the two calls were connected is specified in the request to the next page:
// Unpack the request var telephonyRequest = new TelephonyRequest(Request); var connectResult = (ConnectResult)telephonyRequest.InstanceInfo.ActionResult; double connectedDuration = connectResult.SecondsConnectedDuration;
-
Connect one of two calls, classifying the callee. If it is an answering machine wait for the message to finish but hang up if it's a fax machine:
List<TelephonyAction> actions = new List<TelephonyAction>(); var connectAction = new Connect("443069990123") { CallFrom = "443069999876", HoldMedia = Play.PlayFile("welcome.wav"), SecondsAnswerTimeout = 20, SecondaryCall = new SecondaryCall(null, 60, true), NextPage = new WebPageRequest("ConnectNextPage.aspx") }; var classifyCallee = new ClassifyCallee(3000, false); classifyCallee.ConfigureAnsweringMachineReadyToRecord(new AnsweringMachineReadyToRecord(180, 1000, 4000, 10)); connectAction.EnableCalleeClassification(classifyCallee); connectAction.AddDestination("443069990124"); actions.Add(connectAction);
-
Make two calls, requesting connectFirstPage when the first of the two calls is answered. Connect this call once all connectFirstPage's actions have run. Request connectFinalPage then connectNextPage once the connected call hangs up:
List<TelephonyAction> actions = new List<TelephonyAction>(); var connectAction = new Connect("443069990123") { CallFrom = "443069999876", HoldMedia = Play.PlayFile("welcome.wav"), SecondsAnswerTimeout = 20, SecondaryCall = new SecondaryCall(new WebPageRequest("ConnectFirstPage.aspx"), 60, true) { FinalPage = new WebPageRequest("ConnectFinalPage.aspx"), ErrorPage = new WebPageRequest("ConnectErrorPage.aspx") } }; connectAction.AddDestination("443069990124"); actions.Add(connectAction);
-
Make a call, passing any early media from the secondary call to the primary call:
List<TelephonyAction> actions = new List<TelephonyAction>(); var connectAction = new Connect("443069990123") { CallFrom = "443069999876", PassEarlyMedia = true, SecondaryCall = new SecondaryCall() { CallRecording = true, MinutesMaxCallDuration = 60, FinalPage = new WebPageRequest("ConnectFinalPage.aspx"), ErrorPage = new WebPageRequest("ConnectErrorPage.aspx") }, NextPage = new WebPageRequest("ConnectNextPage.aspx") }; actions.Add(connectAction);
-
Make a call with a translator:
List<TelephonyAction> actions = new List<TelephonyAction>(); // How the primary call handles translation var primaryCallTranslationOptions = new CallTranslationOptions() { SpeechRecognitionOptions = new SpeechRecognitionOptions() { Language = "en-GB", SpeechModelOptions = new SpeechModelOptions(model : "latest_long") }, TTSVoice = "French France Female Polly Lea" }; // How the secondary call handles translation var secondaryCallTranslationOptions = new CallTranslationOptions() { SpeechRecognitionOptions = new SpeechRecognitionOptions() { Language = "fr-FR", SpeechModelOptions = new SpeechModelOptions(model : "phone_call", enhanced : true) }, TTSVoice = "English UK Female Polly Amy" }; var connectAction = new Connect("443069990123") { CallFrom = "443069999876", SecondaryCall = new SecondaryCall() { CallRecording = true, MinutesMaxCallDuration = 60, FinalPage = new WebPageRequest("ConnectFinalPage.aspx"), ErrorPage = new WebPageRequest("ConnectErrorPage.aspx") }, Translator = new Translator(primaryCallTranslationOptions, secondaryCallTranslationOptions) { TranscriptionResultsPage = new WebPageRequest("transcriptionResultsPage.aspx"), Id = "1234" }, NextPage = new WebPageRequest("ConnectNextPage.aspx") }; actions.Add(connectAction);
-
-
public class Connect : TelephonyAction { // Constructors public Connect(string destination); // Members public void AddDestination(string destination); public string CallFrom; public Play HoldMedia; public bool PassEarlyMedia; public int SecondsAnswerTimeout; public void EnableCalleeClassification(); public void EnableCalleeClassification(ClassifyCallee classifyCallee); public SecondaryCall SecondaryCall; public Translator Translator; public WebPageRequest NextPage; }
Examples:
-
Make a call to a single destination, without classifying the callee, and connect it to the existing call:
List<TelephonyAction> actions = new List<TelephonyAction>(); var connectAction = new Connect("443069990123") { CallFrom = "443069999876", NextPage = new WebPageRequest("ConnectNextPage.aspx") }; actions.Add(connectAction);
Once the connect action has finished the duration for which the two calls were connected is specified in the request to the next page:
// Unpack the request var telephonyRequest = await TelephonyRequest.UnpackRequestAsync(Request); var connectResult = (ConnectResult)telephonyRequest.InstanceInfo.ActionResult; double connectedDuration = connectResult.SecondsConnectedDuration;
-
Connect one of two calls, classifying the callee. If it is an answering machine wait for the message to finish but hang up if it's a fax machine:
List<TelephonyAction> actions = new List<TelephonyAction>(); var connectAction = new Connect("443069990123") { CallFrom = "443069999876", HoldMedia = Play.PlayFile("welcome.wav"), SecondsAnswerTimeout = 20, SecondaryCall = new SecondaryCall(null, 60, true), NextPage = new WebPageRequest("ConnectNextPage.aspx") }; var classifyCallee = new ClassifyCallee(3000, false); classifyCallee.ConfigureAnsweringMachineReadyToRecord(new AnsweringMachineReadyToRecord(180, 1000, 4000, 10)); connectAction.EnableCalleeClassification(classifyCallee); connectAction.AddDestination("443069990124"); actions.Add(connectAction);
-
Make two calls, requesting connectFirstPage when the first of the two calls is answered. Connect this call once all connectFirstPage's actions have run. Request connectFinalPage then connectNextPage once the connected call hangs up:
List<TelephonyAction> actions = new List<TelephonyAction>(); var connectAction = new Connect("443069990123") { CallFrom = "443069999876", HoldMedia = Play.PlayFile("welcome.wav"), SecondsAnswerTimeout = 20, SecondaryCall = new SecondaryCall(new WebPageRequest("ConnectFirstPage.aspx"), 60, true) { FinalPage = new WebPageRequest("ConnectFinalPage.aspx"), ErrorPage = new WebPageRequest("ConnectErrorPage.aspx") } }; connectAction.AddDestination("443069990124"); actions.Add(connectAction);
-
Make a call, passing any early media from the secondary call to the primary call:
List<TelephonyAction> actions = new List<TelephonyAction>(); var connectAction = new Connect("443069990123") { CallFrom = "443069999876", PassEarlyMedia = true, SecondaryCall = new SecondaryCall() { CallRecording = true, MinutesMaxCallDuration = 60, FinalPage = new WebPageRequest("ConnectFinalPage.aspx"), ErrorPage = new WebPageRequest("ConnectErrorPage.aspx") }, NextPage = new WebPageRequest("ConnectNextPage.aspx") }; actions.Add(connectAction);
-
Make a call with a translator:
List<TelephonyAction> actions = new List<TelephonyAction>(); // How the primary call handles translation var primaryCallTranslationOptions = new CallTranslationOptions() { SpeechRecognitionOptions = new SpeechRecognitionOptions() { Language = "en-GB", SpeechModelOptions = new SpeechModelOptions(model : "latest_long") }, TTSVoice = "French France Female Polly Lea" }; // How the secondary call handles translation var secondaryCallTranslationOptions = new CallTranslationOptions() { SpeechRecognitionOptions = new SpeechRecognitionOptions() { Language = "fr-FR", SpeechModelOptions = new SpeechModelOptions(model : "phone_call", enhanced : true) }, TTSVoice = "English UK Female Polly Amy" }; var connectAction = new Connect("443069990123") { CallFrom = "443069999876", SecondaryCall = new SecondaryCall() { CallRecording = true, MinutesMaxCallDuration = 60, FinalPage = new WebPageRequest("ConnectFinalPage.aspx"), ErrorPage = new WebPageRequest("ConnectErrorPage.aspx") }, Translator = new Translator(primaryCallTranslationOptions, secondaryCallTranslationOptions) { TranscriptionResultsPage = new WebPageRequest("transcriptionResultsPage.aspx"), Id = "1234" }, NextPage = new WebPageRequest("ConnectNextPage.aspx") }; actions.Add(connectAction);
-
-
-
Connect Class
Namespace: Aculab.Cloud.RestAPIWrapper
Assembly: Aculab.Cloud.RestAPIWrapper.dllAn action to connect an existing inbound or outbound (primary) call to a new (secondary) call destination.
-
Public Class Connect Inherits TelephonyAction ' Constructors Public Sub New (destination As String) ' Members Public Function AddDestination(destination As String) As Void Public Property CallFrom As String Public Property HoldMedia As Play Public Property PassEarlyMedia As Bool Public Property SecondsAnswerTimeout As Integer Public Function EnableCalleeClassification() As Void Public Function EnableCalleeClassification(classifyCallee As Classifycallee) As Void Public Property SecondaryCall As Secondarycall Public Property Translator As Translator Public Property NextPage As Webpagerequest End Class
Examples:
-
Make a call to a single destination, without classifying the callee, and connect it to the existing call:
Dim actions = New List(Of TelephonyAction) Dim connectAction = New Connect("443069990123") With { .CallFrom = "443069999876", .NextPage = New WebPageRequest("ConnectNextPage.aspx") } actions.Add(connectAction)
Once the connect action has finished the duration for which the two calls were connected Is specified in the request to the next page.
' Unpack the request Dim telephonyRequest = New TelephonyRequest(Request) Dim connectResult As ConnectResult = telephonyRequest.InstanceInfo.ActionResult Dim connectedDuration = connectResult.SecondsConnectedDuration
-
Connect one of two calls, classifying the callee. If it is an answering machine wait for the message to finish but hang up if it's a fax machine:
Dim actions = New List(Of TelephonyAction) Dim connectAction = New Connect("443069990123") With { .CallFrom = "443069999876", .HoldMedia = Play.PlayFile("welcome.wav"), .SecondsAnswerTimeout = 20, .SecondaryCall = New SecondaryCall(Nothing, 60, True), .NextPage = New WebPageRequest("ConnectNextPage.aspx") } connectAction.AddDestination("443069990124") Dim classifyCallee = New ClassifyCallee(3000, False) classifyCallee.ConfigureAnsweringMachineReadyToRecord(New AnsweringMachineReadyToRecord(180, 1000, 4000, 10)) connectAction.EnableCalleeClassification(classifyCallee) actions.Add(connectAction)
-
Make two calls, requesting connectFirstPage when the first of the two calls is answered. Connect this call once all connectFirstPage's actions have run. Request connectFinalPage then connectNextPage once the connected call hangs up:
Dim actions = New List(Of TelephonyAction) Dim connectAction = New Connect("443069990123") With { .CallFrom = "443069999876", .HoldMedia = Play.PlayFile("welcome.wav"), .SecondsAnswerTimeout = 20, .SecondaryCall = New SecondaryCall(New WebPageRequest("ConnectFirstPage.aspx"), 60, True) With { .FinalPage = New WebPageRequest("ConnectFinalPage.aspx"), .ErrorPage = New WebPageRequest("ConnectErrorPage.aspx") } } connectAction.AddDestination("443069990124") actions.Add(connectAction)
-
Make a call, passing any early media from the secondary call to the primary call:
Dim actions = New List(Of TelephonyAction) Dim connectAction = New Connect("443069990123") With { .CallFrom = "443069999876", .PassEarlyMedia = True, .SecondaryCall = New SecondaryCall With { .CallRecording = True, .MinutesMaxCallDuration = 60, .FinalPage = New WebPageRequest("ConnectFinalPage.aspx"), .ErrorPage = New WebPageRequest("ConnectErrorPage.aspx") }, .NextPage = New WebPageRequest("ConnectNextPage.aspx") } actions.Add(connectAction)
-
Make a call with a translator:
Dim actions = New List(Of TelephonyAction) ' How the primary call handles translation Dim primaryCallTranslationOptions = New CallTranslationOptions With { .SpeechRecognitionOptions = New SpeechRecognitionOptions() With { .Language = "en-GB", .SpeechModelOptions = New SpeechModelOptions(model:="latest_long") }, .TTSVoice = "French France Female Polly Lea" } ' How the secondary call handles translation Dim secondaryCallTranslationOptions = New CallTranslationOptions() With { .SpeechRecognitionOptions = New SpeechRecognitionOptions() With { .Language = "fr-FR", .SpeechModelOptions = New SpeechModelOptions(model:="phone_call", enhanced:=True) }, .TTSVoice = "English UK Female Polly Amy" } Dim connectAction = New Connect("443069990123") With { .CallFrom = "443069999876", .SecondaryCall = New SecondaryCall() With { .CallRecording = True, .MinutesMaxCallDuration = 60, .FinalPage = New WebPageRequest("ConnectFinalPage.aspx"), .ErrorPage = New WebPageRequest("ConnectErrorPage.aspx") }, .Translator = New Translator(primaryCallTranslationOptions, secondaryCallTranslationOptions) With { .TranscriptionResultsPage = New WebPageRequest("transcriptionResultsPage.aspx"), .Id = "1234" }, .NextPage = New WebPageRequest("ConnectNextPage.aspx") } actions.Add(connectAction)
-
-
Public Class Connect Inherits TelephonyAction ' Constructors Public Sub New (destination As String) ' Members Public Function AddDestination(destination As String) As Void Public Property CallFrom As String Public Property HoldMedia As Play Public Property PassEarlyMedia As Bool Public Property SecondsAnswerTimeout As Integer Public Function EnableCalleeClassification() As Void Public Function EnableCalleeClassification(classifyCallee As Classifycallee) As Void Public Property SecondaryCall As Secondarycall Public Property Translator As Translator Public Property NextPage As Webpagerequest End Class
Examples:
-
Make a call to a single destination, without classifying the callee, and connect it to the existing call:
Dim actions = New List(Of TelephonyAction) Dim connectAction = New Connect("443069990123") With { .CallFrom = "443069999876", .NextPage = New WebPageRequest("ConnectNextPage.aspx") } actions.Add(connectAction)
Once the connect action has finished the duration for which the two calls were connected Is specified in the request to the next page.
' Unpack the request Dim telephonyRequest = New TelephonyRequest(Request) Dim connectResult As ConnectResult = telephonyRequest.InstanceInfo.ActionResult Dim connectedDuration = connectResult.SecondsConnectedDuration
-
Connect one of two calls, classifying the callee. If it is an answering machine wait for the message to finish but hang up if it's a fax machine:
Dim actions = New List(Of TelephonyAction) Dim connectAction = New Connect("443069990123") With { .CallFrom = "443069999876", .HoldMedia = Play.PlayFile("welcome.wav"), .SecondsAnswerTimeout = 20, .SecondaryCall = New SecondaryCall(Nothing, 60, True), .NextPage = New WebPageRequest("ConnectNextPage.aspx") } connectAction.AddDestination("443069990124") Dim classifyCallee = New ClassifyCallee(3000, False) classifyCallee.ConfigureAnsweringMachineReadyToRecord(New AnsweringMachineReadyToRecord(180, 1000, 4000, 10)) connectAction.EnableCalleeClassification(classifyCallee) actions.Add(connectAction)
-
Make two calls, requesting connectFirstPage when the first of the two calls is answered. Connect this call once all connectFirstPage's actions have run. Request connectFinalPage then connectNextPage once the connected call hangs up:
Dim actions = New List(Of TelephonyAction) Dim connectAction = New Connect("443069990123") With { .CallFrom = "443069999876", .HoldMedia = Play.PlayFile("welcome.wav"), .SecondsAnswerTimeout = 20, .SecondaryCall = New SecondaryCall(New WebPageRequest("ConnectFirstPage.aspx"), 60, True) With { .FinalPage = New WebPageRequest("ConnectFinalPage.aspx"), .ErrorPage = New WebPageRequest("ConnectErrorPage.aspx") } } connectAction.AddDestination("443069990124") actions.Add(connectAction)
-
Make a call, passing any early media from the secondary call to the primary call:
Dim actions = New List(Of TelephonyAction) Dim connectAction = New Connect("443069990123") With { .CallFrom = "443069999876", .PassEarlyMedia = True, .SecondaryCall = New SecondaryCall With { .CallRecording = True, .MinutesMaxCallDuration = 60, .FinalPage = New WebPageRequest("ConnectFinalPage.aspx"), .ErrorPage = New WebPageRequest("ConnectErrorPage.aspx") }, .NextPage = New WebPageRequest("ConnectNextPage.aspx") } actions.Add(connectAction)
-
Make a call with a translator:
Dim actions = New List(Of TelephonyAction) ' How the primary call handles translation Dim primaryCallTranslationOptions = New CallTranslationOptions With { .SpeechRecognitionOptions = New SpeechRecognitionOptions() With { .Language = "en-GB", .SpeechModelOptions = New SpeechModelOptions(model:="latest_long") }, .TTSVoice = "French France Female Polly Lea" } ' How the secondary call handles translation Dim secondaryCallTranslationOptions = New CallTranslationOptions() With { .SpeechRecognitionOptions = New SpeechRecognitionOptions() With { .Language = "fr-FR", .SpeechModelOptions = New SpeechModelOptions(model:="phone_call", enhanced:=True) }, .TTSVoice = "English UK Female Polly Amy" } Dim connectAction = New Connect("443069990123") With { .CallFrom = "443069999876", .SecondaryCall = New SecondaryCall() With { .CallRecording = True, .MinutesMaxCallDuration = 60, .FinalPage = New WebPageRequest("ConnectFinalPage.aspx"), .ErrorPage = New WebPageRequest("ConnectErrorPage.aspx") }, .Translator = New Translator(primaryCallTranslationOptions, secondaryCallTranslationOptions) With { .TranscriptionResultsPage = New WebPageRequest("transcriptionResultsPage.aspx"), .Id = "1234" }, .NextPage = New WebPageRequest("ConnectNextPage.aspx") } actions.Add(connectAction)
-
-
-
class Connect extends TelephonyAction
Represents a connect action.
Class synopsis:
// Constructors: public Connect(String destination) // Members: public void addDestination(String destination) public void setCallFrom(String callFrom) public void setHoldMedia(Play holdMedia) public void setPassEarlyMedia(boolean enabled) public void setSecondsAnswerTimeout(int secondsTimeout) public void enableCalleeClassification() public void enableCalleeClassification(ClassifyCallee classifyCallee) public void setSecondaryCall(SecondaryCall secondaryCall) public void setTranslator(Translator translator) public void setNextPage(WebPageRequest nextPage)
class ConnectResult extends ActionResult
Represents the result of a connect action.
Class synopsis:
// Members: public double getSecondsConnectedDuration()
Examples:
Make a call to a single destination, without classifying the callee, and connect it to the existing call:
List<TelephonyAction> actions = new ArrayList<TelephonyAction>(); Connect connectAction = new Connect("443069990123"); connectAction.setCallFrom("443069999876"); connectAction.setNextPage(new WebPageRequest("connectNextPage")); actions.add(connectAction);
Obtain the duration for which the two calls were connected from the action's next page request:
ConnectResult connectResult = (ConnectResult)ourRequest.getInstanceInfo().getActionResult(); double connectedDuration = connectResult.getSecondsConnectedDuration();
Connect one of two calls, classifying the callee. If it is an answering machine wait for the message to finish but hang up if it's a fax machine:
List<TelephonyAction> actions = new ArrayList<TelephonyAction>(); SecondaryCall secCall = new SecondaryCall(); secCall.setCallRecording(true); secCall.setMinutesMaxCallDuration(60); AnsweringMachineReadyToRecord answeringMachineOptions = new AnsweringMachineReadyToRecord(); answeringMachineOptions.setMillisecondsMinBeepDuration(180); answeringMachineOptions.setMillisecondsPostBeepSilence(1000); answeringMachineOptions.setMillisecondsNoBeepSilence(4000); answeringMachineOptions.setSecondsMaxMessageDuration(10); ClassifyCallee classifyCalleeOptions = new ClassifyCallee(); classifyCalleeOptions.setEnable(true); classifyCalleeOptions.setMillisecondsTimeout(3000); classifyCalleeOptions.setHangUpOnAnsweringMachine(false); classifyCalleeOptions.setHangUpOnFaxMachine(true); classifyCalleeOptions.setAnsweringMachineReadyToRecord(answeringMachineOptions); Connect connectAction = new Connect("443069990123"); connectAction.addDestination("443069990124"); connectAction.setCallFrom("443069999876"); connectAction.setHoldMedia(Play.playFile("welcome.wav")); connectAction.setSecondsAnswerTimeout(20); connectAction.setSecondaryCall(secCall); connectAction.enableCalleeClassification(classifyCalleeOptions); connectAction.setNextPage(new WebPageRequest("connectNextPage")); actions.add(connectAction);
Make two calls, requesting connectFirstPage when the first of the two calls is answered. Connect this call once all connectFirstPage's actions have run. Request connectFinalPage then connectNextPage once the connected call hangs up:
List<TelephonyAction> actions = new ArrayList<TelephonyAction>(); SecondaryCall secondaryCall = new SecondaryCall(new WebPageRequest("connectFirstPage"), 60, true); secondaryCall.setFinalPage(new WebPageRequest("connectFinalPage")); secondaryCall.setErrorPage(new WebPageRequest("connectErrorPage")); Connect connectAction = new Connect("443069990123"); connectAction.addDestination("443069990124"); connectAction.setCallFrom("443069999876"); connectAction.setHoldMedia(Play.playFile("welcome.wav")); connectAction.setSecondsAnswerTimeout(20); connectAction.setSecondaryCall(secondaryCall); connectAction.setNextPage(new WebPageRequest("connectNextPage")); actions.add(connectAction);
Make a call, passing any early media from the secondary call to the primary call:
List<TelephonyAction> actions = new ArrayList<TelephonyAction>(); SecondaryCall secondaryCall = new SecondaryCall(); secondaryCall.setCallRecording(true); secondaryCall.setMinutesMaxCallDuration(60); secondaryCall.setFinalPage(new WebPageRequest("connectFinalPage")); secondaryCall.setErrorPage(new WebPageRequest("connectErrorPage")); Connect connectAction = new Connect("443069990123"); connectAction.setCallFrom("443069999876"); connectAction.setPassEarlyMedia(true); connectAction.setSecondaryCall(secondaryCall); connectAction.setNextPage(new WebPageRequest("connectNextPage")); actions.add(connectAction);
Make a call with a translator:
List<TelephonyAction> actions = new ArrayList<TelephonyAction>(); Connect connectAction = new Connect("443069990123"); connectAction.setCallFrom("443069999876"); connectAction.setNextPage(new WebPageRequest("connectNextPage")); { CallTranslationOptions primaryCTO = new CallTranslationOptions(); CallTranslationOptions secondaryCTO = new CallTranslationOptions(); { SpeechModelOptions speechModelOpts = new SpeechModelOptions(); speechModelOpts.setModel("latest_long"); SpeechRecognitionOptions speechRecognitionOpts = new SpeechRecognitionOptions(); speechRecognitionOpts.setLanguage("en-GB"); speechRecognitionOpts.setSpeechModelOptions(speechModelOpts); primaryCTO.setSpeechRecognitionOptions(speechRecognitionOpts); primaryCTO.setTTSVoice("French France Female Polly Lea"); } { SpeechModelOptions speechModelOpts = new SpeechModelOptions(); speechModelOpts.setModel("phone_call"); speechModelOpts.setEnhanced(true); SpeechRecognitionOptions speechRecognitionOpts = new SpeechRecognitionOptions(); speechRecognitionOpts.setLanguage("fr-FR"); speechRecognitionOpts.setSpeechModelOptions(speechModelOpts); secondaryCTO.setSpeechRecognitionOptions(speechRecognitionOpts); secondaryCTO.setTTSVoice("English UK Female Polly Amy"); } WebPageRequest transcriptionResultsPage = new WebPageRequest("transcription_results_page"); Translator translator = new Translator(primaryCTO, secondaryCTO); translator.setTranscriptionResultsPage(transcriptionResultsPage); translator.setID("1234"); connectAction.setTranslator(translator); } actions.add(connectAction);
-
class Connect
Represents a connect action.
Class synopsis:
# Connect object: Connect() # Instance methods: Connect.set_destinations(destinations) Connect.append_destination(destination) Connect.set_call_from(call_from) Connect.set_hold_media(play_action) Connect.set_pass_early_media(pass_early_media=True) Connect.set_seconds_answer_timeout(seconds_answer_timeout) Connect.set_classify_callee(classify_callee) Connect.set_secondary_call(secondary_call) Connect.set_translator(translator) Connect.set_next_page(next_page)
Connect Result
The Connect Result is represented by a dictionary. It is found within the
action result
for thenext page
.Obtaining the Connect Result dictionary:
my_request = TelephonyRequest(request) action_result = my_request.get_action_result() if action_result.get("action") == "connect": connect_result = action_result.get("result")
Examples:
Make a call to a single destination, without classifying the callee, and connect it to the existing call:
# Create a list of actions that will be passed to the TelephonyResponse constructor list_of_actions = [] connect_action = Connect() connect_action.set_destinations(['441908273800']) connect_action.set_call_from('441908273801') connect_action.set_next_page(WebPage(url='connect_next_page')) list_of_actions.append(connect_action)
Obtain the duration for which the two calls were connected from the action's next page request:
my_request = TelephonyRequest(request) action_result = my_request.get_action_result() if action_result.get("action") == "connect": connect_result = action_result.get("result") duration = connect_result.get("seconds_connected_duration") # Your code here...
Connect one of two calls, classifying the callee. If it is an answering machine wait for the message to finish but hang up if it's a fax machine:
# Create a list of actions that will be passed to the TelephonyResponse constructor list_of_actions = [] answering_machine_options = AnsweringMachineReadyToRecord(milliseconds_min_beep_duration=180, milliseconds_post_beep_silence=1000, milliseconds_no_beep_silence=4000, seconds_max_message_duration=10) classify_callee = ClassifyCallee(enable=True, live_speaker_detection_mode='default', hang_up_on_answering_machine=False, hang_up_on_fax_machine=True, answering_machine_ready_to_record=answering_machine_options) connect_action = Connect() connect_action.set_destinations(['441908273800','441908273802']) connect_action.set_call_from('441908273801') connect_action.set_hold_media(Play(file_to_play='welcome.wav')) connect_action.set_seconds_answer_timeout(30) connect_action.set_secondary_call(SecondaryCall(minutes_max_call_duration=240, call_recording=True)) connect_action.set_classify_callee(classify_callee) connect_action.set_next_page(WebPage(url='connect_next_page')) list_of_actions.append(connect_action)
Make two calls, requesting connectFirstPage when the first of the two calls is answered. Connect this call once all connectFirstPage's actions have run. Request connectFinalPage then connectNextPage once the connected call hangs up:
# Create a list of actions that will be passed to the TelephonyResponse constructor list_of_actions = [] connect_action = Connect() connect_action.append_destination('441908273800') connect_action.append_destination('441908273802') connect_action.set_call_from('441908273801') connect_action.set_hold_media(Play(file_to_play='welcome.wav')) connect_action.set_seconds_answer_timeout(30) connect_action.set_secondary_call(SecondaryCall(first_page=WebPage(url='connect_first_page'), final_page=WebPage(url='connect_final_page'), error_page=WebPage(url='connect_error_page'), minutes_max_call_duration=240, call_recording=True)) connect_action.set_next_page(WebPage(url='connect_next_page')) list_of_actions.append(connect_action)
Make a call, passing any early media from the secondary call to the primary call:
# Create a list of actions that will be passed to the TelephonyResponse constructor list_of_actions = [] connect_action = Connect() connect_action.append_destination('441908273800') connect_action.set_call_from('441908273801') connect_action.set_pass_early_media() connect_action.set_secondary_call(SecondaryCall(final_page=WebPage(url='connect_final_page'), error_page=WebPage(url='connect_error_page'), minutes_max_call_duration=240, call_recording=True)) connect_action.set_next_page(WebPage(url='connect_next_page')) list_of_actions.append(connect_action)
Make a call with a translator:
# Create a list of actions that will be passed to the TelephonyResponse constructor list_of_actions = [] connect_action = Connect() connect_action.set_destinations(['441908273800']) connect_action.set_call_from('441908273801') connect_action.set_next_page(WebPage(url='connect_next_page')) primarySMO = SpeechModelOptions() primarySMO.set_model("latest_long") primarySRO = SpeechRecognitionOptions() primarySRO.set_language("en-GB") primarySRO.set_speech_model_options(primarySMO) primaryCTO = CallTranslationOptions() primaryCTO.set_speech_recognition_options(primarySRO) primaryCTO.set_tts_voice("French France Female Polly Lea") secondarySMO = SpeechModelOptions() secondarySMO.set_model("phone_call") secondarySMO.set_enhanced(True) secondarySRO = SpeechRecognitionOptions() secondarySRO.set_language("fr-FR") secondarySRO.set_speech_model_options(secondarySMO) secondaryCTO = CallTranslationOptions() secondaryCTO.set_speech_recognition_options(secondarySRO) secondaryCTO.set_tts_voice("English UK Female Polly Amy") translator = Translator(primaryCTO, secondaryCTO) translator.set_transcription_results_page(WebPage(url='transcription_results_page')) translator.set_id("1234") connect_action.set_translator(translator); list_of_actions.append(connect_action)
-
The Connect class
Introduction
Represents a connect action.
Class synopsis
class Connect extends ConnectBase { /* methods */ public __construct() public self addDestination(string $dest) public self setCallFrom(string $from) public self setClassifyCallee(ClassifyCalleeConfiguration $classify = null) public self setPassEarlyMedia(boolean $enabled) public self setTranslator(Translator $translator = null) /* inherited methods */ public self setHoldMedia(Play $play = null) public self setSecondsAnswerTimeout(int $secs) public self setSecondaryCallConfiguration(SecondaryCallConfiguration $secondary_call_configuration) public self setNextPage(string|WebPageRequest $next_page, string $method = null) }
The ConnectResult class
Introduction
Represents the result of a connect action.
Class synopsis
class ConnectResult extends ActionResult { /* methods */ public float getSecondsConnectedDuration() /* inherited methods */ public string getAction() public boolean getInterrupted() }
Examples:
Make a call to a single destination, without classifying the callee, and connect it to the existing call:
$connect = new Aculab\TelephonyRestAPI\Connect(); $connect->addDestination('443069990123'); $connect->setCallFrom('443069999876'); $connect->setNextPage('connectNextPage'); $response->addAction($connect);
Obtain the duration for which the two calls were connected from the action's next page request:
$info = InstanceInfo::getInstanceInfo(); $connectResult = $info->getActionResult(); $durationConnected = $connectResult->getSecondsConnectedDuration();
Connect one of two calls, classifying the callee. If it is an answering machine wait for the message to finish but hang up if it's a fax machine:
$connect = new Aculab\TelephonyRestAPI\Connect(); $connect->addDestination('443069990123'); $connect->addDestination('443069990124'); $connect->setCallFrom('443069999876'); $connect->setHoldMedia(Aculab\TelephonyRestAPI\Play::playFile('welcome.wav')); $connect->setSecondsAnswerTimeout(20); $connect->setNextPage('connectNextPage'); $call_config = new Aculab\TelephonyRestAPI\SecondaryCallConfiguration(); $call_config->setMinutesMaxCallDuration(60); $call_config->setCallRecording(true); $connect->setSecondaryCallConfiguration($call_config); $classify_configuration = new Aculab\TelephonyRestAPI\ClassifyCalleeConfiguration(); $classify_configuration->setMillisecondsTimeout(3000); $classify_configuration->setHangupOnAnsweringMachine(false); $classify_configuration->setAnsweringMachineReadyToRecordConfiguration(180, 1000, 4000, 10); $connect->setClassifyCallee($classify_configuration); $response->addAction($connect);
Make two calls, requesting connectFirstPage when the first of the two calls is answered. Connect this call once all connectFirstPage's actions have run. Request connectFinalPage then connectNextPage once the connected call hangs up:
$connect = new Aculab\TelephonyRestAPI\Connect(); $connect->addDestination('443069990123'); $connect->addDestination('443069990124'); $connect->setCallFrom('443069999876'); $connect->setHoldMedia(Aculab\TelephonyRestAPI\Play::playFile('welcome.wav')); $connect->setSecondsAnswerTimeout(20); $connect->setNextPage('connectNextPage'); $call_config = new Aculab\TelephonyRestAPI\SecondaryCallConfiguration(); $call_config->setMinutesMaxCallDuration(60); $call_config->setCallRecording(true); $call_config->setFirstPage('connectFirstPage'); $call_config->setErrorPage('connectErrorPage'); $call_config->setFinalPage('connectFinalPage'); $connect->setSecondaryCallConfiguration($call_config); $response->addAction($connect);
Make a call, passing any early media from the secondary call to the primary call:
$connect = new Aculab\TelephonyRestAPI\Connect(); $connect->addDestination('443069990123'); $connect->setCallFrom('443069999876'); $connect->setPassEarlyMedia(true); $connect->setNextPage('connectNextPage'); $call_config = new Aculab\TelephonyRestAPI\SecondaryCallConfiguration(); $call_config->setMinutesMaxCallDuration(60); $call_config->setCallRecording(true); $call_config->setErrorPage('connectErrorPage'); $call_config->setFinalPage('connectFinalPage'); $connect->setSecondaryCallConfiguration($call_config); $response->addAction($connect);
Make a call with a translator:
$connect = new Aculab\TelephonyRestAPI\Connect(); $connect->addDestination('443069990123'); $connect->setCallFrom('443069999876'); $connect->setNextPage('connectNextPage'); $translator = new Aculab\TelephonyRestAPI\Translator(); $primary = new Aculab\TelephonyRestAPI\CallTranslationOptions(); $speech_model_options = new \Aculab\TelephonyRestAPI\SpeechModelOptions(); $speech_model_options->setModel("latest_long"); $speech_recognition_options = new \Aculab\TelephonyRestAPI\SpeechRecognitionOptions(); $speech_recognition_options->setLanguage("en-GB"); $speech_recognition_options->setSpeechModelOptions($speech_model_options); $primary->setSpeechRecognitionOptions($speech_recognition_options); $primary->setTTSVoice("French France Female Polly Lea"); $secondary = new Aculab\TelephonyRestAPI\CallTranslationOptions(); $speech_model_options = new \Aculab\TelephonyRestAPI\SpeechModelOptions(); $speech_model_options->setModel("phone_call") ->setEnhanced(true); $speech_recognition_options = new \Aculab\TelephonyRestAPI\SpeechRecognitionOptions(); $speech_recognition_options->setLanguage("fr-FR"); $speech_recognition_options->setSpeechModelOptions($speech_model_options); $secondary->setSpeechRecognitionOptions($speech_recognition_options); $secondary->setTTSVoice("English UK Female Polly Amy"); $translator->setPrimaryCallTranslationOptions($primary) ->setSecondaryCallTranslationOptions($secondary) ->setTranscriptionResultsPage("transcriptionResultsPage") ->setID("1234"); $connect->setTranslator($translator); $response->addAction($connect);