get input Action
Gets input from the call, either a spoken word or short phrase or entered digits. Optionally it can play a prompt on the call to request input. You can specify hints for words that may be said, and a set of valid digits that may be input. You can also configure get input to retry one or more times by specifying messages to play to the call if nothing is input or invalid digits are entered.
Speech input can be disabled or operate in two modes: timeout and auto. timeout mode allows you to specify a maximum time between spoken words. auto mode is designed to be used for short prompts and short immediate responses.
A get input
action uses one speech recognition stream. The service must be configured so that the application will have one stream available when the action runs. If the prompt play has barge in on speech
enabled, it will share the speech recognition stream.
Note that speech recognition/transcription is a chargeable feature.
language wrappers and examples
The get input properties are:
Property | Required/Optional | Default | Description |
---|---|---|---|
next page | required | A web page request object that defines the web page to be requested once the get input action has completed successfully (some speech or valid digits have been input). The recognised speech or digits entered will be sent to this page. | |
prompt | optional | - | A play action that can prompt for words, phrases or digits. Note that barge in on speech options are ignored for this play action. |
speech input mode from API V2.0 | optional | timeout | A string. One of timeout , disabled or auto .timeout: if set to timeout then milliseconds inter speech timeout controls how long the action waits before deciding there is no more speech input.disabled: if set to disabled then the action does not run speech recognition.auto: if set to auto then milliseconds inter speech timeout is ignored. In certain circumstances this can result in better performance than timeout mode. Please contact us for advice on how to use this mode. |
speech recognition options from API V2.0 | optional | - | A speech recognition options object that sets the language for the call and other options related to speech recognition. |
digit input enabled | optional | true | Whether digit input is enabled. If it is, digit input options determines which digits are valid and how the digit entry is completed. |
digit input options | optional | - | A digit input options object that determines which digits are valid and how to determine whether the digit input is complete. Omitting this results in the default settings for digit input options to be used. |
seconds timeout from API V2.0 | optional | 30 | The time in seconds that the action waits for all input to complete after the prompt has finished. A value of 0 means that the action will return immediately with whatever input is currently available. |
milliseconds inter speech timeout from API V2.0 | optional | 1000 | An integer. The time period in milliseconds that the action waits for each consecutive word. A value of 0 or a negative value signifies that this timeout is disabled. |
milliseconds inter digit timeout from API V2.0 | optional | 3000 | An integer. The time period in milliseconds that the action waits for each consecutive digit to be entered. A value of 0 or a negative value signifies that this timeout is disabled. |
milliseconds initial timeout from API V2.0 | optional | 5000 | An integer. The time period in milliseconds that the action waits for initial speech or digits after the prompt has finished. A value of 0 or a negative value signifies that this timeout is disabled. |
on input timeout messages | optional |
"Sorry, I did not get anything.", "Please listen to the instructions, and then speak clearly or press the relevant digits." |
An array of play actions. This defines messages to play if no speech is recognised or no valid digits are entered within the allotted time. Each play action in the array is played once for each successive timeout. When all messages have been played the get input action finishes. |
on invalid input messages | optional |
"Sorry, that isn't valid input.", "Please listen to the instructions, and then speak clearly or press the relevant digits." |
An array of play actions. This defines messages to play if invalid digits are entered. Each play action in the array is played once for each successive invalid digit entered. When all messages have been played the get input action finishes. Note that all speech input is regarded as valid. |
help digit | optional | "*" | A single digit that, when pressed, will result in the prompt being repeated. An empty string signifies no help digit. Valid digits are 0123456789ABCD#*. |
Remarks
Digit input takes precedence:
At any point, if digits are detected, any transcribed speech will be ignored.
Speech input modes:
- timeout mode:
is designed to be used when prompts are long and speech input is expected to be long.
In this mode you can specify the maximum period without recognising further speech after which the action deems speech input to have finished (
milliseconds inter speech timeout
). - auto mode:
is designed to be used when prompts and responses are short and made without significant delay.
milliseconds inter speech timeout
is ignored in this mode.
In timeout mode:
With no barge in - milliseconds initial timeout
is applied after the prompt has finished until the first speech is recognised. get input returns if milliseconds inter speech timeout
expires before the next word/phrase is recognised. seconds timeout
is measured from the end of the prompt.
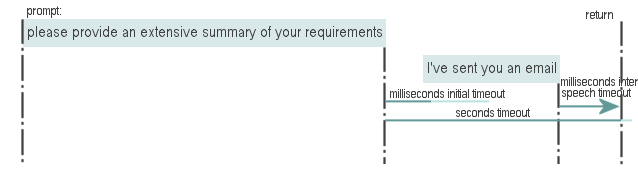
With barge in - Once speech has been recognised the prompt stops and get input returns if either milliseconds inter speech timeout
expires before the next word/phrase is recognised or seconds timeout
expires.
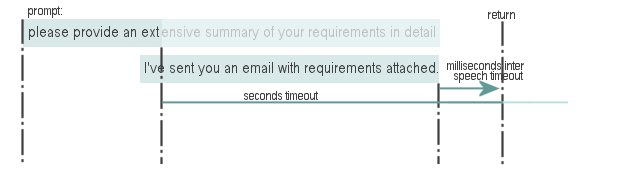
In auto mode:
With no barge in - milliseconds initial timeout
is applied after the prompt has finished until the first speech is recognised. get input returns after a phrase of speech is recognised or
if seconds timeout
expires.
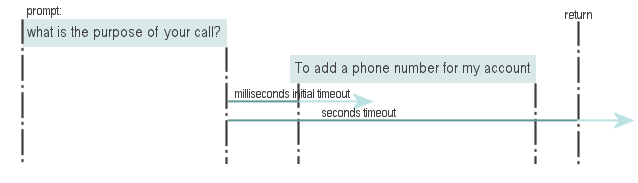
With barge in - Once speech has been recognised the prompt stops and get input returns after a phrase of speech is recognised or if seconds timeout
expires.
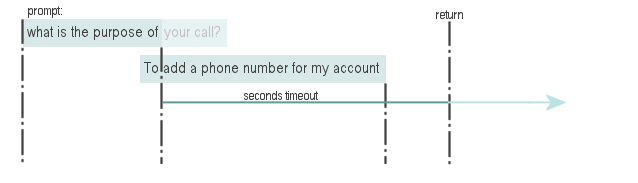
Getting speech or digits from a preceding play action:
It inspects any digits or speech that may have already been cached from barge in on a preceding play action.
If the play barge in occurred due to speech then get_input
returns that speech immediately. If the barge in was due to a digit it prefixes any further digits entered. In this case, a prompt
that has barge in on digit
enabled will not be played.
To avoid this behaviour you can insert a clear input action after the play action.
Returns
The result of the get input action will be returned via the subsequent HTTP Request to the next page
in the action result as follows:
Property | Availability | Description |
---|---|---|
speech input | if input type is 'speech' | If input type is 'speech' this will contain an array of phrase objects representing the recognised speech. |
digits input | if input type is 'digits' | If input type is 'digits' this will contain a string consisting of the digits entered (not including the end digit ). |
input type | always | A string indicating how the input was obtained. Either 'speech' or 'digits'. |
If no speech or valid digits are input within seconds timeout
and after any configured retries, this action does not request the next page
and drops through to the next action in the current list.
-
Examples:
Get a single utterance of speech:
"get_input" : { "prompt" : { "play" : { "play_list" : [ { "text_to_say" : "Please say the reason for your call." } ] } }, "digit_input_enabled" : false, "next_page" : { "url" : "useinputpage" } }
The following may be returned:
"action_result" : { "action" : "get_input", "result" : { "input_type": "speech", "speech_input" : [ { "alternatives" : [ { "text" : "I'm calling to close my account", "confidence" : 0.89 } ], "direction" : "inbound", "final" : true } ] } }
Get a name, specifying some likely hints, allowing speech barge in while the prompt is playing, but disabling digit input and barge in:
"get_input" : { "prompt" : { "play" : { "play_list" : [ { "text_to_say" : "Please say the name of a 17th century author." } ], "barge_in_on_speech" : true, "barge_in_on_digit" : false } }, "digit_input_enabled" : false, "speech_recognition_options" : { "word_hints" : [ { "words": [ "Alexander Pope", "Cyrano de Bergerac", "Daniel Defoe", "John Milton", "Jonathan Swift", "Voltaire" ] } ] }, "next_page" : { "url" : "useinputpage" } }
The following may be returned:
"action_result" : { "action" : "get_input", "result" : { "input_type": "speech", "speech_input" : [ { "alternatives" : [ { "text" : "jonathan swift", "confidence" : 0.92 } ], "direction" : "inbound", "final" : true } ] } }
or this:
"action_result" : { "action" : "get_input", "result" : { "input_type": "speech", "speech_input" : [ { "alternatives" : [ { "text" : "william shakespeare", "confidence" : 0.87 } ], "direction" : "inbound", "final" : true } ] } }
Get the name of a month or the month number as entered digits, setting the inter digit timeout:
"get_input" : { "prompt" : { "play" : { "play_list" : [ { "text_to_say" : "Please say a month name or enter the month number." } ] } }, "milliseconds_inter_digit_timeout" : 1500, "next_page" : { "url" : "useinputpage" } }
The following may be returned when 'March' is said:
"action_result" : { "action" : "get_input", "result" : { "input_type": "speech", "speech_input" : [ { "alternatives" : [ { "text" : "March", "confidence" : 0.92 } ], "direction" : "inbound", "final" : true } ] } }
The following may be returned if the digit 3 is pressed followed by a '#':
"action_result" : { "action" : "get_input", "result" : { "input_type": "digits", "digits_input" : "3" } }
Get a single digit number either spoken or as an entered digit:
"get_input" : { "prompt" : { "play" : { "play_list" : [ { "text_to_say" : "Please say a single number from zero to nine, or press a single digit." } ] } }, "digit_input_options" : { "digit_count" : 1 }, "next_page" : { "url" : "useinputpage" } }
The following may be returned when 'seven' is said:
"action_result" : { "action" : "get_input", "result" : { "input_type": "speech", "speech_input" : [ { "alternatives" : [ { "text" : "7", "confidence" : 0.89 } ], "direction" : "inbound", "final" : true } ] } }
The following may be returned if the digit 7 is pressed:
"action_result" : { "action" : "get_input", "result" : { "input_type": "digits", "digits_input" : "7" } }
Get an eight digit number either spoken or as entered digits, specifying longer timeouts for speech input:
"get_input" : { "prompt" : { "play" : { "play_list" : [ { "text_to_say" : "Please say the 8 digit account number or enter it on your keypad." } ] } }, "digit_input_options" : { "digit_count" : 8 }, "milliseconds_inter_speech_timeout" : 2000, "milliseconds_initial_timeout" : 10000, "next_page" : { "url" : "useinputpage" } }
The following may be returned:
"action_result" : { "action" : "get_input", "result" : { "input_type": "speech", "speech_input" : [ { "alternatives" : [ { "text" : "58190229", "confidence" : 0.91 } ], "direction" : "inbound", "final" : true } ] } }
The following may be returned if keypad digits are pressed:
"action_result" : { "action" : "get_input", "result" : { "input_type": "digits", "digits_input" : "58190229" } }
Get a three digit number only consisting of the digits 1-5:
"get_input" : { "prompt" : { "play" : { "play_list" : [ { "text_to_say" : "Please say three digits from one to five." } ] } }, "speech_input_mode" : "auto", "digit_input_options" : { "digit_count" : 3, "valid_digits" : "12345" }, "on_invalid_input_messages" : [ { "play" : { "play_list" : [ { "text_to_say" : "Please enter only digits from one to five." } ] } }, { "play" : { "play_list" : [ { "text_to_say" : "No really!" }, { "text_to_say" : "Please enter only digits from one to five." } ] } } ], "next_page" : { "url" : "useinputpage" } }
The following may be returned:
"action_result" : { "action" : "get_input", "result" : { "input_type": "speech", "speech_input" : [ { "alternatives" : [ { "text" : "625", "confidence" : 0.88 } ], "direction" : "inbound", "final" : true } ] } }
Get an arbitrary length number and set custom retry messages:
"get_input" : { "prompt" : { "play" : { "play_list" : [ { "text_to_say" : "Please say your membership number." } ] } }, "digit_input_enabled" : false, "on_input_timeout_messages" : [ { "play" : { "play_list" : [ { "file_to_play" : "silencemessage1.wav" } ] } }, { "play" : { "play_list" : [ { "file_to_play" : "silencemessage2.wav" } ] } } ], "next_page" : { "url" : "useinputpage" } }
The following may be returned:
"action_result" : { "action" : "get_input", "result" : { "input_type": "speech", "speech_input" : [ { "alternatives" : [ { "text" : "0061425000", "confidence" : 0.93 } ], "direction" : "inbound", "final" : true } ] } }
-
GetInput Class
Namespace: Aculab.Cloud.RestAPIWrapper
Assembly: Aculab.Cloud.RestAPIWrapper.dllThe speech input mode that determines whether speech input is enabled and, if so, whether timeouts are set manually or automatically.
-
public class GetInput : TelephonyAction { // Constructors public GetInput(WebPageRequest nextPage, Play prompt = null, SpeechRecognitionOptions options = null); // Members public WebPageRequest NextPage; public Play Prompt; public SpeechInputMode SpeechInputMode; public SpeechRecognitionOptions SpeechRecognitionOptions; public bool DigitInputEnabled; public DigitInputOptions DigitInputOptions; public int SecondsTimeout; public int MillisecondsInterSpeechTimeout; public int MillisecondsInterDigitTimeout; public int MillisecondsInitialTimeout; public List<Play> OnInputTimeoutMessages; public List<Play> OnInvalidInputMessages; public char? HelpDigit; }
Examples:
-
Get a single utterance of speech:
List<TelephonyAction> actions = new List<TelephonyAction>(); var prompt = Play.SayText("Please say the reason for your call."); var getInputAction = new GetInput(new WebPageRequest("UseInputPage.aspx"), prompt); getInputAction.DigitInputEnabled = false; actions.Add(getInputAction);
Extract the speech from the action result:
// Unpack the request var telephonyRequest = new TelephonyRequest(Request); var getInputResult = (GetInputResult)telephonyRequest.InstanceInfo.ActionResult; if (getInputResult.SpeechInput.Equals("speech")) { foreach (Phrase phrase in getInputResult.SpeechInput) { // Here, we are just using the first alternative var speech = phrase.Alternatives[0]; var text = speech.Text; var confidence = speech.Confidence; // Handle spoken phrase... } }
-
Get a name, specifying some likely hints, allowing speech barge in while the prompt is playing, but disabling digit input and barge in:
List<TelephonyAction> actions = new List<TelephonyAction>(); var prompt = Play.SayText("Please say the name of a 17th century author."); prompt.BargeInOnSpeech = true; prompt.BargeInOnDigit = false; var getInputAction = new GetInput(new WebPageRequest("UseInputPage.aspx"), prompt); getInputAction.DigitInputEnabled = false; var options = new SpeechRecognitionOptions(); var hint = new WordHint("Alexander Pope"); hint.Words.Add("Cyrano de Bergerac"); hint.Words.Add("Daniel Defoe"); hint.Words.Add("John Milton"); hint.Words.Add("Jonathan Swift"); hint.Words.Add("Voltaire"); options.WordHints.Add(hint); getInputAction.SpeechRecognitionOptions = options; actions.Add(getInputAction);
Extract the spoken phrase from the action result:
// Unpack the request var telephonyRequest = new TelephonyRequest(Request); var getInputResult = (GetInputResult)telephonyRequest.InstanceInfo.ActionResult; if (getInputResult.SpeechInput.Equals("speech")) { foreach (Phrase phrase in getInputResult.SpeechInput) { // Here, we are just using the first alternative var speech = phrase.Alternatives[0]; var text = speech.Text; var confidence = speech.Confidence; // Handle spoken phrase... } }
-
Get the name of a month or the month number as entered digits, setting the inter digit timeout:
List<TelephonyAction> actions = new List<TelephonyAction>(); var prompt = Play.SayText("Please say a month name or enter the month number."); var getInputAction = new GetInput(new WebPageRequest("UseInputPage.aspx"), prompt); getInputAction.MillisecondsInterDigitTimeout = 1500; actions.Add(getInputAction);
Extract the input from the action result:
// Unpack the request var telephonyRequest = new TelephonyRequest(Request); var getInputResult = (GetInputResult)telephonyRequest.InstanceInfo.ActionResult; if (getInputResult.SpeechInput.Equals("speech")) { foreach (Phrase phrase in getInputResult.SpeechInput) { // Here, we are just using the first alternative var speech = phrase.Alternatives[0]; var text = speech.Text; var confidence = speech.Confidence; // Handle spoken phrase... } } else { var digits = getInputResult.DigitsInput; // Handle digit input... }
-
Get a single digit number either spoken or as an entered digit:
List<TelephonyAction> actions = new List<TelephonyAction>(); var prompt = Play.SayText("Please say a single number from zero to nine, or press a single digit."); var getInputAction = new GetInput(new WebPageRequest("UseInputPage.aspx"), prompt); var digitOptions = new DigitInputOptions(1); getInputAction.DigitInputOptions = digitOptions; actions.Add(getInputAction);
Extract the input from the action result:
// Unpack the request var telephonyRequest = new TelephonyRequest(Request); var getInputResult = (GetInputResult)telephonyRequest.InstanceInfo.ActionResult; if (getInputResult.SpeechInput.Equals("speech")) { foreach (Phrase phrase in getInputResult.SpeechInput) { // Here, we are just using the first alternative var speech = phrase.Alternatives[0]; var text = speech.Text; var confidence = speech.Confidence; // Handle spoken phrase... } } else { var digit = getInputResult.DigitsInput; // Handle digit input... }
-
Get an eight digit number either spoken or as entered digits, specifying longer timeouts for speech input:
List<TelephonyAction> actions = new List<TelephonyAction>(); var prompt = Play.SayText("Please say the 8 digit account number or enter it on your keypad."); var getInputAction = new GetInput(new WebPageRequest("UseInputPage.aspx"), prompt); getInputAction.MillisecondsInterSpeechTimeout = 2000; getInputAction.MillisecondsInitialTimeout = 10000; var digitOptions = new DigitInputOptions(8); getInputAction.DigitInputOptions = digitOptions; actions.Add(getInputAction);
Extract the input from the action result:
// Unpack the request var telephonyRequest = new TelephonyRequest(Request); var getInputResult = (GetInputResult)telephonyRequest.InstanceInfo.ActionResult; if (getInputResult.SpeechInput.Equals("speech")) { foreach (Phrase phrase in getInputResult.SpeechInput) { // Here, we are just using the first alternative var speech = phrase.Alternatives[0]; var text = speech.Text; var confidence = speech.Confidence; // Handle spoken phrase... } } else { var digits = getInputResult.DigitsInput; // Handle digit input... }
-
Get a three digit number only consisting of the digits 1-5:
List<TelephonyAction> actions = new List<TelephonyAction>(); var prompt = Play.SayText("Please say three digits from one to five."); var getInputAction = new GetInput(new WebPageRequest("UseInputPage.aspx"), prompt); getInputAction.SpeechInputMode = SpeechInputMode.Auto; var digitOptions = new DigitInputOptions(3); digitOptions.ValidDigits = "12345"; getInputAction.DigitInputOptions = digitOptions; var invalidInputMessages = new List<Play>(); invalidInputMessages.Add(Play.SayText("Please enter only digits from one to five.")); var play = Play.SayText("No really!"); play.AddText("Please enter only digits from one to five."); invalidInputMessages.Add(play); getInputAction.OnInvalidInputMessages = invalidInputMessages; actions.Add(getInputAction);
Extract the input from the action result:
// Unpack the request var telephonyRequest = new TelephonyRequest(Request); var getInputResult = (GetInputResult)telephonyRequest.InstanceInfo.ActionResult; if (getInputResult.SpeechInput.Equals("speech")) { foreach (Phrase phrase in getInputResult.SpeechInput) { // Here, we are just using the first alternative var speech = phrase.Alternatives[0]; var text = speech.Text; var confidence = speech.Confidence; // Handle spoken phrase... } } else { var digits = getInputResult.DigitsInput; // Handle digit input... }
-
Get an arbitrary length number and set custom retry messages:
List<TelephonyAction> actions = new List<TelephonyAction>(); var prompt = Play.SayText("Please say your membership number."); var getInputAction = new GetInput(new WebPageRequest("UseInputPage.aspx"), prompt); getInputAction.DigitInputEnabled = false; var inputTimeoutMessages = new List<Play>(); inputTimeoutMessages.Add(Play.PlayFile("silencemessage1.wav")); inputTimeoutMessages.Add(Play.PlayFile("silencemessage2.wav")); getInputAction.OnInputTimeoutMessages = inputTimeoutMessages; actions.Add(getInputAction);
Extract the speech from the action result:
// Unpack the request var telephonyRequest = new TelephonyRequest(Request); var getInputResult = (GetInputResult)telephonyRequest.InstanceInfo.ActionResult; if (getInputResult.SpeechInput.Equals("speech")) { foreach (Phrase phrase in getInputResult.SpeechInput) { // Here, we are just using the first alternative var speech = phrase.Alternatives[0]; var text = speech.Text; var confidence = speech.Confidence; // Handle spoken phrase... } }
-
-
public class GetInput : TelephonyAction { // Constructors public GetInput(WebPageRequest nextPage, Play prompt = null, SpeechRecognitionOptions options = null); // Members public WebPageRequest NextPage; public Play Prompt; public SpeechInputMode SpeechInputMode; public SpeechRecognitionOptions SpeechRecognitionOptions; public bool DigitInputEnabled; public DigitInputOptions DigitInputOptions; public int SecondsTimeout; public int MillisecondsInterSpeechTimeout; public int MillisecondsInterDigitTimeout; public int MillisecondsInitialTimeout; public List<Play> OnInputTimeoutMessages; public List<Play> OnInvalidInputMessages; public char? HelpDigit; }
Examples:
-
Get a single utterance of speech:
List<TelephonyAction> actions = new List<TelephonyAction>(); var prompt = Play.SayText("Please say the reason for your call."); var getInputAction = new GetInput(new WebPageRequest("UseInputPage.aspx"), prompt); getInputAction.DigitInputEnabled = false; actions.Add(getInputAction);
Extract the speech from the action result:
// Unpack the request var telephonyRequest = new TelephonyRequest(Request); var getInputResult = (GetInputResult)telephonyRequest.InstanceInfo.ActionResult; if (getInputResult.SpeechInput.Equals("speech")) { foreach (Phrase phrase in getInputResult.SpeechInput) { // Here, we are just using the first alternative var speech = phrase.Alternatives[0]; var text = speech.Text; var confidence = speech.Confidence; // Handle spoken phrase... } }
-
Get a name, specifying some likely hints, allowing speech barge in while the prompt is playing, but disabling digit input and barge in:
List<TelephonyAction> actions = new List<TelephonyAction>(); var prompt = Play.SayText("Please say the name of a 17th century author."); prompt.BargeInOnSpeech = true; prompt.BargeInOnDigit = false; var getInputAction = new GetInput(new WebPageRequest("UseInputPage.aspx"), prompt); getInputAction.DigitInputEnabled = false; var options = new SpeechRecognitionOptions(); var hint = new WordHint("Alexander Pope"); hint.Words.Add("Cyrano de Bergerac"); hint.Words.Add("Daniel Defoe"); hint.Words.Add("John Milton"); hint.Words.Add("Jonathan Swift"); hint.Words.Add("Voltaire"); options.WordHints.Add(hint); getInputAction.SpeechRecognitionOptions = options; actions.Add(getInputAction);
Extract the spoken phrase from the action result:
// Unpack the request var telephonyRequest = new TelephonyRequest(Request); var getInputResult = (GetInputResult)telephonyRequest.InstanceInfo.ActionResult; if (getInputResult.SpeechInput.Equals("speech")) { foreach (Phrase phrase in getInputResult.SpeechInput) { // Here, we are just using the first alternative var speech = phrase.Alternatives[0]; var text = speech.Text; var confidence = speech.Confidence; // Handle spoken phrase... } }
-
Get the name of a month or the month number as entered digits, setting the inter digit timeout:
List<TelephonyAction> actions = new List<TelephonyAction>(); var prompt = Play.SayText("Please say a month name or enter the month number."); var getInputAction = new GetInput(new WebPageRequest("UseInputPage.aspx"), prompt); getInputAction.MillisecondsInterDigitTimeout = 1500; actions.Add(getInputAction);
Extract the input from the action result:
// Unpack the request var telephonyRequest = new TelephonyRequest(Request); var getInputResult = (GetInputResult)telephonyRequest.InstanceInfo.ActionResult; if (getInputResult.SpeechInput.Equals("speech")) { foreach (Phrase phrase in getInputResult.SpeechInput) { // Here, we are just using the first alternative var speech = phrase.Alternatives[0]; var text = speech.Text; var confidence = speech.Confidence; // Handle spoken phrase... } } else { var digits = getInputResult.DigitsInput; // Handle digit input... }
-
Get a single digit number either spoken or as an entered digit:
List<TelephonyAction> actions = new List<TelephonyAction>(); var prompt = Play.SayText("Please say a single number from zero to nine, or press a single digit."); var getInputAction = new GetInput(new WebPageRequest("UseInputPage.aspx"), prompt); var digitOptions = new DigitInputOptions(1); getInputAction.DigitInputOptions = digitOptions; actions.Add(getInputAction);
Extract the input from the action result:
// Unpack the request var telephonyRequest = new TelephonyRequest(Request); var getInputResult = (GetInputResult)telephonyRequest.InstanceInfo.ActionResult; if (getInputResult.SpeechInput.Equals("speech")) { foreach (Phrase phrase in getInputResult.SpeechInput) { // Here, we are just using the first alternative var speech = phrase.Alternatives[0]; var text = speech.Text; var confidence = speech.Confidence; // Handle spoken phrase... } } else { var digit = getInputResult.DigitsInput; // Handle digit input... }
-
Get an eight digit number either spoken or as entered digits, specifying longer timeouts for speech input:
List<TelephonyAction> actions = new List<TelephonyAction>(); var prompt = Play.SayText("Please say the 8 digit account number or enter it on your keypad."); var getInputAction = new GetInput(new WebPageRequest("UseInputPage.aspx"), prompt); getInputAction.MillisecondsInterSpeechTimeout = 2000; getInputAction.MillisecondsInitialTimeout = 10000; var digitOptions = new DigitInputOptions(8); getInputAction.DigitInputOptions = digitOptions; actions.Add(getInputAction);
Extract the input from the action result:
// Unpack the request var telephonyRequest = new TelephonyRequest(Request); var getInputResult = (GetInputResult)telephonyRequest.InstanceInfo.ActionResult; if (getInputResult.SpeechInput.Equals("speech")) { foreach (Phrase phrase in getInputResult.SpeechInput) { // Here, we are just using the first alternative var speech = phrase.Alternatives[0]; var text = speech.Text; var confidence = speech.Confidence; // Handle spoken phrase... } } else { var digits = getInputResult.DigitsInput; // Handle digit input... }
-
Get a three digit number only consisting of the digits 1-5:
List<TelephonyAction> actions = new List<TelephonyAction>(); var prompt = Play.SayText("Please say three digits from one to five."); var getInputAction = new GetInput(new WebPageRequest("UseInputPage.aspx"), prompt); getInputAction.SpeechInputMode = SpeechInputMode.Auto; var digitOptions = new DigitInputOptions(3); digitOptions.ValidDigits = "12345"; getInputAction.DigitInputOptions = digitOptions; var invalidInputMessages = new List<Play>(); invalidInputMessages.Add(Play.SayText("Please enter only digits from one to five.")); var play = Play.SayText("No really!"); play.AddText("Please enter only digits from one to five."); invalidInputMessages.Add(play); getInputAction.OnInvalidInputMessages = invalidInputMessages; actions.Add(getInputAction);
Extract the input from the action result:
// Unpack the request var telephonyRequest = new TelephonyRequest(Request); var getInputResult = (GetInputResult)telephonyRequest.InstanceInfo.ActionResult; if (getInputResult.SpeechInput.Equals("speech")) { foreach (Phrase phrase in getInputResult.SpeechInput) { // Here, we are just using the first alternative var speech = phrase.Alternatives[0]; var text = speech.Text; var confidence = speech.Confidence; // Handle spoken phrase... } } else { var digits = getInputResult.DigitsInput; // Handle digit input... }
-
Get an arbitrary length number and set custom retry messages:
List<TelephonyAction> actions = new List<TelephonyAction>(); var prompt = Play.SayText("Please say your membership number."); var getInputAction = new GetInput(new WebPageRequest("UseInputPage.aspx"), prompt); getInputAction.DigitInputEnabled = false; var inputTimeoutMessages = new List<Play>(); inputTimeoutMessages.Add(Play.PlayFile("silencemessage1.wav")); inputTimeoutMessages.Add(Play.PlayFile("silencemessage2.wav")); getInputAction.OnInputTimeoutMessages = inputTimeoutMessages; actions.Add(getInputAction);
Extract the speech from the action result:
// Unpack the request var telephonyRequest = new TelephonyRequest(Request); var getInputResult = (GetInputResult)telephonyRequest.InstanceInfo.ActionResult; if (getInputResult.SpeechInput.Equals("speech")) { foreach (Phrase phrase in getInputResult.SpeechInput) { // Here, we are just using the first alternative var speech = phrase.Alternatives[0]; var text = speech.Text; var confidence = speech.Confidence; // Handle spoken phrase... } }
-
-
public class GetInput : TelephonyAction { // Constructors public GetInput(WebPageRequest nextPage, Play prompt = null, SpeechRecognitionOptions options = null); // Members public WebPageRequest NextPage; public Play Prompt; public SpeechInputMode SpeechInputMode; public SpeechRecognitionOptions SpeechRecognitionOptions; public bool DigitInputEnabled; public DigitInputOptions DigitInputOptions; public int SecondsTimeout; public int MillisecondsInterSpeechTimeout; public int MillisecondsInterDigitTimeout; public int MillisecondsInitialTimeout; public List<Play> OnInputTimeoutMessages; public List<Play> OnInvalidInputMessages; public char? HelpDigit; }
Examples:
-
Get a single utterance of speech:
List<TelephonyAction> actions = new List<TelephonyAction>(); var prompt = Play.SayText("Please say the reason for your call."); var getInputAction = new GetInput(new WebPageRequest("UseInputPage.aspx"), prompt); getInputAction.DigitInputEnabled = false; actions.Add(getInputAction);
Extract the speech from the action result:
// Unpack the request var telephonyRequest = await TelephonyRequest.UnpackRequestAsync(Request); var getInputResult = (GetInputResult)telephonyRequest.InstanceInfo.ActionResult; if (getInputResult.SpeechInput.Equals("speech")) { foreach (Phrase phrase in getInputResult.SpeechInput) { // Here, we are just using the first alternative var speech = phrase.Alternatives[0]; var text = speech.Text; var confidence = speech.Confidence; // Handle spoken phrase... } }
-
Get a name, specifying some likely hints, allowing speech barge in while the prompt is playing, but disabling digit input and barge in:
List<TelephonyAction> actions = new List<TelephonyAction>(); var prompt = Play.SayText("Please say the name of a 17th century author."); prompt.BargeInOnSpeech = true; prompt.BargeInOnDigit = false; var getInputAction = new GetInput(new WebPageRequest("UseInputPage.aspx"), prompt); getInputAction.DigitInputEnabled = false; var options = new SpeechRecognitionOptions(); var hint = new WordHint("Alexander Pope"); hint.Words.Add("Cyrano de Bergerac"); hint.Words.Add("Daniel Defoe"); hint.Words.Add("John Milton"); hint.Words.Add("Jonathan Swift"); hint.Words.Add("Voltaire"); options.WordHints.Add(hint); getInputAction.SpeechRecognitionOptions = options; actions.Add(getInputAction);
Extract the spoken phrase from the action result:
// Unpack the request var telephonyRequest = await TelephonyRequest.UnpackRequestAsync(Request); var getInputResult = (GetInputResult)telephonyRequest.InstanceInfo.ActionResult; if (getInputResult.SpeechInput.Equals("speech")) { foreach (Phrase phrase in getInputResult.SpeechInput) { // Here, we are just using the first alternative var speech = phrase.Alternatives[0]; var text = speech.Text; var confidence = speech.Confidence; // Handle spoken phrase... } }
-
Get the name of a month or the month number as entered digits, setting the inter digit timeout:
List<TelephonyAction> actions = new List<TelephonyAction>(); var prompt = Play.SayText("Please say a month name or enter the month number."); var getInputAction = new GetInput(new WebPageRequest("UseInputPage.aspx"), prompt); getInputAction.MillisecondsInterDigitTimeout = 1500; actions.Add(getInputAction);
Extract the input from the action result:
// Unpack the request var telephonyRequest = await TelephonyRequest.UnpackRequestAsync(Request); var getInputResult = (GetInputResult)telephonyRequest.InstanceInfo.ActionResult; if (getInputResult.SpeechInput.Equals("speech")) { foreach (Phrase phrase in getInputResult.SpeechInput) { // Here, we are just using the first alternative var speech = phrase.Alternatives[0]; var text = speech.Text; var confidence = speech.Confidence; // Handle spoken phrase... } } else { var digits = getInputResult.DigitsInput; // Handle digit input... }
-
Get a single digit number either spoken or as an entered digit:
List<TelephonyAction> actions = new List<TelephonyAction>(); var prompt = Play.SayText("Please say a single number from zero to nine, or press a single digit."); var getInputAction = new GetInput(new WebPageRequest("UseInputPage.aspx"), prompt); var digitOptions = new DigitInputOptions(1); getInputAction.DigitInputOptions = digitOptions; actions.Add(getInputAction);
Extract the input from the action result:
// Unpack the request var telephonyRequest = await TelephonyRequest.UnpackRequestAsync(Request); var getInputResult = (GetInputResult)telephonyRequest.InstanceInfo.ActionResult; if (getInputResult.SpeechInput.Equals("speech")) { foreach (Phrase phrase in getInputResult.SpeechInput) { // Here, we are just using the first alternative var speech = phrase.Alternatives[0]; var text = speech.Text; var confidence = speech.Confidence; // Handle spoken phrase... } } else { var digit = getInputResult.DigitsInput; // Handle digit input... }
-
Get an eight digit number either spoken or as entered digits, specifying longer timeouts for speech input:
List<TelephonyAction> actions = new List<TelephonyAction>(); var prompt = Play.SayText("Please say the 8 digit account number or enter it on your keypad."); var getInputAction = new GetInput(new WebPageRequest("UseInputPage.aspx"), prompt); getInputAction.MillisecondsInterSpeechTimeout = 2000; getInputAction.MillisecondsInitialTimeout = 10000; var digitOptions = new DigitInputOptions(8); getInputAction.DigitInputOptions = digitOptions; actions.Add(getInputAction);
Extract the input from the action result:
// Unpack the request var telephonyRequest = await TelephonyRequest.UnpackRequestAsync(Request); var getInputResult = (GetInputResult)telephonyRequest.InstanceInfo.ActionResult; if (getInputResult.SpeechInput.Equals("speech")) { foreach (Phrase phrase in getInputResult.SpeechInput) { // Here, we are just using the first alternative var speech = phrase.Alternatives[0]; var text = speech.Text; var confidence = speech.Confidence; // Handle spoken phrase... } } else { var digits = getInputResult.DigitsInput; // Handle digit input... }
-
Get a three digit number only consisting of the digits 1-5:
List<TelephonyAction> actions = new List<TelephonyAction>(); var prompt = Play.SayText("Please say three digits from one to five."); var getInputAction = new GetInput(new WebPageRequest("UseInputPage.aspx"), prompt); getInputAction.SpeechInputMode = SpeechInputMode.Auto; var digitOptions = new DigitInputOptions(3); digitOptions.ValidDigits = "12345"; getInputAction.DigitInputOptions = digitOptions; var invalidInputMessages = new List<Play>(); invalidInputMessages.Add(Play.SayText("Please enter only digits from one to five.")); var play = Play.SayText("No really!"); play.AddText("Please enter only digits from one to five."); invalidInputMessages.Add(play); getInputAction.OnInvalidInputMessages = invalidInputMessages; actions.Add(getInputAction);
Extract the input from the action result:
// Unpack the request var telephonyRequest = await TelephonyRequest.UnpackRequestAsync(Request); var getInputResult = (GetInputResult)telephonyRequest.InstanceInfo.ActionResult; if (getInputResult.SpeechInput.Equals("speech")) { foreach (Phrase phrase in getInputResult.SpeechInput) { // Here, we are just using the first alternative var speech = phrase.Alternatives[0]; var text = speech.Text; var confidence = speech.Confidence; // Handle spoken phrase... } } else { var digits = getInputResult.DigitsInput; // Handle digit input... }
-
Get an arbitrary length number and set custom retry messages:
List<TelephonyAction> actions = new List<TelephonyAction>(); var prompt = Play.SayText("Please say your membership number."); var getInputAction = new GetInput(new WebPageRequest("UseInputPage.aspx"), prompt); getInputAction.DigitInputEnabled = false; var inputTimeoutMessages = new List<Play>(); inputTimeoutMessages.Add(Play.PlayFile("silencemessage1.wav")); inputTimeoutMessages.Add(Play.PlayFile("silencemessage2.wav")); getInputAction.OnInputTimeoutMessages = inputTimeoutMessages; actions.Add(getInputAction);
Extract the speech from the action result:
// Unpack the request var telephonyRequest = await TelephonyRequest.UnpackRequestAsync(Request); var getInputResult = (GetInputResult)telephonyRequest.InstanceInfo.ActionResult; if (getInputResult.SpeechInput.Equals("speech")) { foreach (Phrase phrase in getInputResult.SpeechInput) { // Here, we are just using the first alternative var speech = phrase.Alternatives[0]; var text = speech.Text; var confidence = speech.Confidence; // Handle spoken phrase... } }
-
-
-
GetInput Class
Namespace: Aculab.Cloud.RestAPIWrapper
Assembly: Aculab.Cloud.RestAPIWrapper.dllThe speech input mode that determines whether speech input is enabled and, if so, whether timeouts are set manually or automatically.
-
Public Class GetInput Inherits TelephonyAction ' Constructors Public Sub New (nextPage As Webpagerequest, Optional prompt As Play = Nothing, Optional options As Speechrecognitionoptions = Nothing) ' Members Public Property NextPage As Webpagerequest Public Property Prompt As Play Public Property SpeechInputMode As Speechinputmode Public Property SpeechRecognitionOptions As Speechrecognitionoptions Public Property DigitInputEnabled As Bool Public Property DigitInputOptions As Digitinputoptions Public Property SecondsTimeout As Integer Public Property MillisecondsInterSpeechTimeout As Integer Public Property MillisecondsInterDigitTimeout As Integer Public Property MillisecondsInitialTimeout As Integer Public Property OnInputTimeoutMessages As List(Of Play) Public Property OnInvalidInputMessages As List(Of Play) Public Property HelpDigit As Char? End Class
Examples:
-
Get a single utterance of speech:
Dim actions = New List(Of TelephonyAction) Dim prompt = Play.SayText("Please say the reason for your call.") Dim getInputAction = New GetInput(New WebPageRequest("UseInputPage.aspx"), prompt) getInputAction.DigitInputEnabled = False actions.Add(getInputAction)
Extract the speech from the action result:
' Unpack the request Dim TelephonyRequest = New TelephonyRequest(Request) Dim GetInputResult As GetInputResult = TelephonyRequest.InstanceInfo.ActionResult If GetInputResult.SpeechInput.Equals("speech") Then For Each phrase In GetInputResult.SpeechInput ' Here, we are just using the first alternative Dim Speech = phrase.Alternatives(0) Dim Text = Speech.Text Dim confidence = Speech.Confidence ' Handle spoken phrase... Next End If
-
Get a name, specifying some likely hints, allowing speech barge in while the prompt is playing, but disabling digit input and barge in:
Dim actions = New List(Of TelephonyAction) Dim prompt = Play.SayText("Please say the name of a 17th century author.") prompt.BargeInOnSpeech = True prompt.BargeInOnDigit = False Dim getInputAction = New GetInput(New WebPageRequest("UseInputPage.aspx"), prompt) getInputAction.DigitInputEnabled = False Dim options = New SpeechRecognitionOptions() Dim hint = New WordHint("Alexander Pope") hint.Words.Add("Cyrano de Bergerac") hint.Words.Add("Daniel Defoe") hint.Words.Add("John Milton") hint.Words.Add("Jonathan Swift") hint.Words.Add("Voltaire") options.WordHints.Add(hint) getInputAction.SpeechRecognitionOptions = options actions.Add(getInputAction)
Extract the spoken phrase from the action result:
' Unpack the request Dim TelephonyRequest = New TelephonyRequest(Request) Dim GetInputResult As GetInputResult = TelephonyRequest.InstanceInfo.ActionResult If GetInputResult.SpeechInput.Equals("speech") Then For Each phrase In GetInputResult.SpeechInput ' Here, we are just using the first alternative Dim Speech = phrase.Alternatives(0) Dim Text = Speech.Text Dim confidence = Speech.Confidence ' Handle spoken phrase... Next End If
-
Get the name of a month or the month number as entered digits, setting the inter digit timeout:
Dim actions = New List(Of TelephonyAction) Dim prompt = Play.SayText("Please say a month name or enter the month number.") Dim getInputAction = New GetInput(New WebPageRequest("UseInputPage.aspx"), prompt) getInputAction.MillisecondsInterDigitTimeout = 1500 actions.Add(getInputAction)
Extract the input from the action result:
' Unpack the request Dim TelephonyRequest = New TelephonyRequest(Request) Dim GetInputResult As GetInputResult = TelephonyRequest.InstanceInfo.ActionResult If GetInputResult.SpeechInput.Equals("speech") Then For Each phrase In GetInputResult.SpeechInput ' Here, we are just using the first alternative Dim Speech = phrase.Alternatives(0) Dim Text = Speech.Text Dim confidence = Speech.Confidence ' Handle spoken phrase... Next Else Dim digits = GetInputResult.DigitsInput ' Handle digit input... End If
-
Get a single digit number either spoken or as an entered digit:
Dim actions = New List(Of TelephonyAction) Dim prompt = Play.SayText("Please say a single number from zero to nine, or press a single digit.") Dim getInputAction = New GetInput(New WebPageRequest("UseInputPage.aspx"), prompt) Dim digitOptions = New DigitInputOptions(1) getInputAction.DigitInputOptions = digitOptions actions.Add(getInputAction)
Extract the input from the action result:
' Unpack the request Dim TelephonyRequest = New TelephonyRequest(Request) Dim GetInputResult As GetInputResult = TelephonyRequest.InstanceInfo.ActionResult If GetInputResult.SpeechInput.Equals("speech") Then For Each phrase In GetInputResult.SpeechInput ' Here, we are just using the first alternative Dim Speech = phrase.Alternatives(0) Dim Text = Speech.Text Dim confidence = Speech.Confidence ' Handle spoken phrase... Next Else Dim digit = GetInputResult.DigitsInput ' Handle digit input... End If
-
Get an eight digit number either spoken or as entered digits, specifying longer timeouts for speech input:
Dim actions = New List(Of TelephonyAction) Dim prompt = Play.SayText("Please say the 8 digit account number or enter it on your keypad.") Dim getInputAction = New GetInput(New WebPageRequest("UseInputPage.aspx"), prompt) getInputAction.MillisecondsInterSpeechTimeout = 2000 getInputAction.MillisecondsInitialTimeout = 10000 Dim digitOptions = New DigitInputOptions(8) getInputAction.DigitInputOptions = digitOptions actions.Add(getInputAction)
Extract the input from the action result:
' Unpack the request Dim TelephonyRequest = New TelephonyRequest(Request) Dim GetInputResult As GetInputResult = TelephonyRequest.InstanceInfo.ActionResult If GetInputResult.SpeechInput.Equals("speech") Then For Each phrase In GetInputResult.SpeechInput ' Here, we are just using the first alternative Dim Speech = phrase.Alternatives(0) Dim Text = Speech.Text Dim confidence = Speech.Confidence ' Handle spoken phrase... Next Else Dim digits = GetInputResult.DigitsInput ' Handle digit input... End If
-
Get a three digit number only consisting of the digits 1-5:
Dim actions = New List(Of TelephonyAction) Dim prompt = Play.SayText("Please say three digits from one to five.") Dim getInputAction = New GetInput(New WebPageRequest("UseInputPage.aspx"), prompt) getInputAction.SpeechInputMode = SpeechInputMode.Auto Dim digitOptions = New DigitInputOptions(3) digitOptions.ValidDigits = "12345" getInputAction.DigitInputOptions = digitOptions Dim invalidInputMessages = New List(Of Play) invalidInputMessages.Add(Play.SayText("Please enter only digits from one to five.")) Dim message = Play.SayText("No really!") message.AddText("Please enter only digits from one to five.") invalidInputMessages.Add(message) getInputAction.OnInvalidInputMessages = invalidInputMessages actions.Add(getInputAction)
Extract the input from the action result:
' Unpack the request Dim TelephonyRequest = New TelephonyRequest(Request) Dim GetInputResult As GetInputResult = TelephonyRequest.InstanceInfo.ActionResult If GetInputResult.SpeechInput.Equals("speech") Then For Each phrase In GetInputResult.SpeechInput ' Here, we are just using the first alternative Dim Speech = phrase.Alternatives(0) Dim Text = Speech.Text Dim confidence = Speech.Confidence ' Handle spoken phrase... Next Else Dim digits = GetInputResult.DigitsInput ' Handle digit input... End If
-
Get an arbitrary length number and set custom retry messages:
Dim actions = New List(Of TelephonyAction) Dim prompt = Play.SayText("Please say your membership number.") Dim getInputAction = New GetInput(New WebPageRequest("UseInputPage.aspx"), prompt) getInputAction.DigitInputEnabled = False Dim inputTimeoutMessages = New List(Of Play) inputTimeoutMessages.Add(Play.PlayFile("silencemessage1.wav")) inputTimeoutMessages.Add(Play.PlayFile("silencemessage2.wav")) getInputAction.OnInputTimeoutMessages = inputTimeoutMessages actions.Add(getInputAction)
Extract the speech from the action result:
' Unpack the request Dim telephonyRequest = New TelephonyRequest(Request) Dim GetInputResult As GetInputResult = telephonyRequest.InstanceInfo.ActionResult If GetInputResult.SpeechInput.Equals("speech") Then For Each phrase In GetInputResult.SpeechInput ' Here, we are just using the first alternative Dim speech = phrase.Alternatives(0) Dim text = speech.Text Dim confidence = speech.Confidence ' Handle spoken phrase... Next End If
-
-
Public Class GetInput Inherits TelephonyAction ' Constructors Public Sub New (nextPage As Webpagerequest, Optional prompt As Play = Nothing, Optional options As Speechrecognitionoptions = Nothing) ' Members Public Property NextPage As Webpagerequest Public Property Prompt As Play Public Property SpeechInputMode As Speechinputmode Public Property SpeechRecognitionOptions As Speechrecognitionoptions Public Property DigitInputEnabled As Bool Public Property DigitInputOptions As Digitinputoptions Public Property SecondsTimeout As Integer Public Property MillisecondsInterSpeechTimeout As Integer Public Property MillisecondsInterDigitTimeout As Integer Public Property MillisecondsInitialTimeout As Integer Public Property OnInputTimeoutMessages As List(Of Play) Public Property OnInvalidInputMessages As List(Of Play) Public Property HelpDigit As Char? End Class
Examples:
-
Get a single utterance of speech:
Dim actions = New List(Of TelephonyAction) Dim prompt = Play.SayText("Please say the reason for your call.") Dim getInputAction = New GetInput(New WebPageRequest("UseInputPage.aspx"), prompt) getInputAction.DigitInputEnabled = False actions.Add(getInputAction)
Extract the speech from the action result:
' Unpack the request Dim TelephonyRequest = New TelephonyRequest(Request) Dim GetInputResult As GetInputResult = TelephonyRequest.InstanceInfo.ActionResult If GetInputResult.SpeechInput.Equals("speech") Then For Each phrase In GetInputResult.SpeechInput ' Here, we are just using the first alternative Dim Speech = phrase.Alternatives(0) Dim Text = Speech.Text Dim confidence = Speech.Confidence ' Handle spoken phrase... Next End If
-
Get a name, specifying some likely hints, allowing speech barge in while the prompt is playing, but disabling digit input and barge in:
Dim actions = New List(Of TelephonyAction) Dim prompt = Play.SayText("Please say the name of a 17th century author.") prompt.BargeInOnSpeech = True prompt.BargeInOnDigit = False Dim getInputAction = New GetInput(New WebPageRequest("UseInputPage.aspx"), prompt) getInputAction.DigitInputEnabled = False Dim options = New SpeechRecognitionOptions() Dim hint = New WordHint("Alexander Pope") hint.Words.Add("Cyrano de Bergerac") hint.Words.Add("Daniel Defoe") hint.Words.Add("John Milton") hint.Words.Add("Jonathan Swift") hint.Words.Add("Voltaire") options.WordHints.Add(hint) getInputAction.SpeechRecognitionOptions = options actions.Add(getInputAction)
Extract the spoken phrase from the action result:
' Unpack the request Dim TelephonyRequest = New TelephonyRequest(Request) Dim GetInputResult As GetInputResult = TelephonyRequest.InstanceInfo.ActionResult If GetInputResult.SpeechInput.Equals("speech") Then For Each phrase In GetInputResult.SpeechInput ' Here, we are just using the first alternative Dim Speech = phrase.Alternatives(0) Dim Text = Speech.Text Dim confidence = Speech.Confidence ' Handle spoken phrase... Next End If
-
Get the name of a month or the month number as entered digits, setting the inter digit timeout:
Dim actions = New List(Of TelephonyAction) Dim prompt = Play.SayText("Please say a month name or enter the month number.") Dim getInputAction = New GetInput(New WebPageRequest("UseInputPage.aspx"), prompt) getInputAction.MillisecondsInterDigitTimeout = 1500 actions.Add(getInputAction)
Extract the input from the action result:
' Unpack the request Dim TelephonyRequest = New TelephonyRequest(Request) Dim GetInputResult As GetInputResult = TelephonyRequest.InstanceInfo.ActionResult If GetInputResult.SpeechInput.Equals("speech") Then For Each phrase In GetInputResult.SpeechInput ' Here, we are just using the first alternative Dim Speech = phrase.Alternatives(0) Dim Text = Speech.Text Dim confidence = Speech.Confidence ' Handle spoken phrase... Next Else Dim digits = GetInputResult.DigitsInput ' Handle digit input... End If
-
Get a single digit number either spoken or as an entered digit:
Dim actions = New List(Of TelephonyAction) Dim prompt = Play.SayText("Please say a single number from zero to nine, or press a single digit.") Dim getInputAction = New GetInput(New WebPageRequest("UseInputPage.aspx"), prompt) Dim digitOptions = New DigitInputOptions(1) getInputAction.DigitInputOptions = digitOptions actions.Add(getInputAction)
Extract the input from the action result:
' Unpack the request Dim TelephonyRequest = New TelephonyRequest(Request) Dim GetInputResult As GetInputResult = TelephonyRequest.InstanceInfo.ActionResult If GetInputResult.SpeechInput.Equals("speech") Then For Each phrase In GetInputResult.SpeechInput ' Here, we are just using the first alternative Dim Speech = phrase.Alternatives(0) Dim Text = Speech.Text Dim confidence = Speech.Confidence ' Handle spoken phrase... Next Else Dim digit = GetInputResult.DigitsInput ' Handle digit input... End If
-
Get an eight digit number either spoken or as entered digits, specifying longer timeouts for speech input:
Dim actions = New List(Of TelephonyAction) Dim prompt = Play.SayText("Please say the 8 digit account number or enter it on your keypad.") Dim getInputAction = New GetInput(New WebPageRequest("UseInputPage.aspx"), prompt) getInputAction.MillisecondsInterSpeechTimeout = 2000 getInputAction.MillisecondsInitialTimeout = 10000 Dim digitOptions = New DigitInputOptions(8) getInputAction.DigitInputOptions = digitOptions actions.Add(getInputAction)
Extract the input from the action result:
' Unpack the request Dim TelephonyRequest = New TelephonyRequest(Request) Dim GetInputResult As GetInputResult = TelephonyRequest.InstanceInfo.ActionResult If GetInputResult.SpeechInput.Equals("speech") Then For Each phrase In GetInputResult.SpeechInput ' Here, we are just using the first alternative Dim Speech = phrase.Alternatives(0) Dim Text = Speech.Text Dim confidence = Speech.Confidence ' Handle spoken phrase... Next Else Dim digits = GetInputResult.DigitsInput ' Handle digit input... End If
-
Get a three digit number only consisting of the digits 1-5:
Dim actions = New List(Of TelephonyAction) Dim prompt = Play.SayText("Please say three digits from one to five.") Dim getInputAction = New GetInput(New WebPageRequest("UseInputPage.aspx"), prompt) getInputAction.SpeechInputMode = SpeechInputMode.Auto Dim digitOptions = New DigitInputOptions(3) digitOptions.ValidDigits = "12345" getInputAction.DigitInputOptions = digitOptions Dim invalidInputMessages = New List(Of Play) invalidInputMessages.Add(Play.SayText("Please enter only digits from one to five.")) Dim message = Play.SayText("No really!") message.AddText("Please enter only digits from one to five.") invalidInputMessages.Add(message) getInputAction.OnInvalidInputMessages = invalidInputMessages actions.Add(getInputAction)
Extract the input from the action result:
' Unpack the request Dim TelephonyRequest = New TelephonyRequest(Request) Dim GetInputResult As GetInputResult = TelephonyRequest.InstanceInfo.ActionResult If GetInputResult.SpeechInput.Equals("speech") Then For Each phrase In GetInputResult.SpeechInput ' Here, we are just using the first alternative Dim Speech = phrase.Alternatives(0) Dim Text = Speech.Text Dim confidence = Speech.Confidence ' Handle spoken phrase... Next Else Dim digits = GetInputResult.DigitsInput ' Handle digit input... End If
-
Get an arbitrary length number and set custom retry messages:
Dim actions = New List(Of TelephonyAction) Dim prompt = Play.SayText("Please say your membership number.") Dim getInputAction = New GetInput(New WebPageRequest("UseInputPage.aspx"), prompt) getInputAction.DigitInputEnabled = False Dim inputTimeoutMessages = New List(Of Play) inputTimeoutMessages.Add(Play.PlayFile("silencemessage1.wav")) inputTimeoutMessages.Add(Play.PlayFile("silencemessage2.wav")) getInputAction.OnInputTimeoutMessages = inputTimeoutMessages actions.Add(getInputAction)
Extract the speech from the action result:
' Unpack the request Dim telephonyRequest = New TelephonyRequest(Request) Dim GetInputResult As GetInputResult = telephonyRequest.InstanceInfo.ActionResult If GetInputResult.SpeechInput.Equals("speech") Then For Each phrase In GetInputResult.SpeechInput ' Here, we are just using the first alternative Dim speech = phrase.Alternatives(0) Dim text = speech.Text Dim confidence = speech.Confidence ' Handle spoken phrase... Next End If
-
-
-
class GetInput extends TelephonyAction
Represents a get input action.
Class synopsis:
// Constructors: public GetInput(WebPageRequest nextPage) public GetInput(WebPageRequest nextPage, Play prompt) // Members: public void setPrompt(Play prompt) public void setSecondsTimeout(int timout) public void setMillisecondsInterSpeechTimeout(int timout) public void setMillisecondsInterDigitTimeout(int timout) public void setMillisecondsInitialTimeout(int timout) public void setDigitInputEnabled(boolean enabled) public void setDigitInputOptions(DigitInputOptions options) public void setSpeechRecognitionOptions(SpeechRecognitionOptions options) public void setSpeechInputMode(SpeechInputMode mode) public void setOnInputTimeoutMessages(List<Play> messageList) public void setOnInvalidInputMessages(List<Play> messageList) public void setHelpDigit(char helpDigit) public JSONObject toJSONObject()
class GetInputResult extends ActionResult
Represents the result of a get input action.
Class synopsis:
// Members: public String getInputType() public String getDigitsInput() public List<Phrase> getSpeechInput()
Examples:
-
Get a single utterance of speech:
List<TelephonyAction> actions = new ArrayList<TelephonyAction>(); GetInput getInputAction = new GetInput(new WebPageRequest("useinputpage")); getInputAction.setPrompt(Play.sayText("Please say the reason for your call.")); getInputAction.setDigitInputEnabled(false); actions.add(getInputAction);
Process the response:
GetInputResult inputResult = (GetInputResult)ourRequest.getInstanceInfo().getActionResult(); if (inputResult.getInputType().equals("speech")) { List<Phrase> phrase_list = inputResult.getSpeechInput(); List<Speech> speech_list = phrase_list.get(0).getAlternatives(); String input = speech_list.get(0).getText(); input = input.toLowerCase(); if(input.contains("close") && input.contains("account")) { // Caller wants to close an account // Your code here... } }
-
Get a name, specifying some likely hints, allowing speech barge in while the prompt is playing, but disabling digit input and barge in:
List<TelephonyAction> actions = new ArrayList<TelephonyAction>(); Play playAction = Play.sayText("Please say the name of a 17th century author."); playAction.setBargeInOnSpeech(true); playAction.setBargeInOnDigit(false); List<String> words = new ArrayList<>(); words.add("Alexander Pope"); words.add("Cyrano de Bergerac"); words.add("Daniel Defoe"); words.add("John Milton"); words.add("Jonathan Swift"); words.add("Voltaire"); WordHint wordHint = new WordHint(words); List<WordHint> wordHints = new ArrayList<>(); wordHints.add(wordHint); SpeechRecognitionOptions speechOptions = new SpeechRecognitionOptions(); speechOptions.setWordHints(wordHints); GetInput getInputAction = new GetInput(new WebPageRequest("useinputpage")); getInputAction.setDigitInputEnabled(false); getInputAction.setSpeechRecognitionOptions(speechOptions); actions.add(getInputAction);
Process the response:
GetInputResult inputResult = (GetInputResult)ourRequest.getInstanceInfo().getActionResult(); if (inputResult.getInputType().equals("speech")) { List<Phrase> phrase_list = inputResult.getSpeechInput(); List<Speech> speech_list = phrase_list.get(0).getAlternatives(); String input = speech_list.get(0).getText(); input = input.toLowerCase(); if(input.contains("jonathan swift")) { // Caller said 'Jonathan Swift' // Your code here... } }
-
Get the name of a month or the month number as entered digits, setting the inter digit timeout:
List<TelephonyAction> actions = new ArrayList<TelephonyAction>(); Play playAction = Play.sayText("Please say a month name or enter the month number."); GetInput getInputAction = new GetInput(new WebPageRequest("useinputpage")); getInputAction.setMillisecondsInterDigitTimeout(1500); getInputAction.setPrompt(playAction); actions.add(getInputAction);
Process the response:
GetInputResult inputResult = (GetInputResult)ourRequest.getInstanceInfo().getActionResult(); if (inputResult.getInputType().equals("speech")) { List<Phrase> phrase_list = inputResult.getSpeechInput(); List<Speech> speech_list = phrase_list.get(0).getAlternatives(); String input = speech_list.get(0).getText(); input = input.toLowerCase(); if(input.equals("march")) { // Caller said 'March' // Your code here... } } else if (inputResult.getInputType().equals("digits")) { String input = inputResult.getDigitsInput(); if(input.equals("3")) { // Caller pressed digit 3 on their keypad. // Your code here... } }
-
Get a single digit number either spoken or as an entered digit:
List<TelephonyAction> actions = new ArrayList<TelephonyAction>(); Play prompt = Play.sayText("Please say a single number from zero to nine, or press a single digit."); DigitInputOptions digitOptions = new DigitInputOptions(1); GetInput getInputAction = new GetInput(new WebPageRequest("useinputpage")); getInputAction.setPrompt(prompt); getInputAction.setDigitInputOptions(digitOptions); actions.add(getInputAction);
Process the response:
GetInputResult inputResult = (GetInputResult)ourRequest.getInstanceInfo().getActionResult(); if (inputResult.getInputType().equals("speech")) { List<Phrase> phrase_list = inputResult.getSpeechInput(); List<Speech> speech_list = phrase_list.get(0).getAlternatives(); String input = speech_list.get(0).getText(); if(input.equals("7")) { // Caller said 'Seven' // Your code here... } } else if (inputResult.getInputType().equals("digits")) { String input = inputResult.getDigitsInput(); if(input.equals("7")) { // Caller pressed digit 7 on their keypad. // Your code here... } }
-
Get an eight digit number either spoken or as entered digits, specifying longer timeouts for speech input:
List<TelephonyAction> actions = new ArrayList<TelephonyAction>(); Play prompt = Play.sayText("Please say the 8 digit account number or enter it on your keypad."); DigitInputOptions digitOptions = new DigitInputOptions(8); GetInput getInputAction = new GetInput(new WebPageRequest("useinputpage")); getInputAction.setMillisecondsInterSpeechTimeout(2000); getInputAction.setMillisecondsInitialTimeout(10000); getInputAction.setPrompt(prompt); getInputAction.setDigitInputOptions(digitOptions); actions.add(getInputAction);
Process the response:
GetInputResult inputResult = (GetInputResult)ourRequest.getInstanceInfo().getActionResult(); if (inputResult.getInputType().equals("speech")) { List<Phrase> phrase_list = inputResult.getSpeechInput(); List<Speech> speech_list = phrase_list.get(0).getAlternatives(); String input = speech_list.get(0).getText(); // Your code here... } else if (inputResult.getInputType().equals("digits")) { String input = inputResult.getDigitsInput(); // Your code here... }
-
Get a three digit number only consisting of the digits 1-5:
List<TelephonyAction> actions = new ArrayList<TelephonyAction>(); Play prompt = Play.sayText("Please say three digits from one to five."); DigitInputOptions digitOptions = new DigitInputOptions(3); digitOptions.setValidDigits("12345"); List<Play> onInvalidInputMessages = new ArrayList<Play>(); onInvalidInputMessages.add(Play.sayText("Please enter only digits from one to five.")); Play finalMessage = Play.sayText("No really!"); finalMessage.addText("Please enter only digits from one to five."); onInvalidInputMessages.add(finalMessage); GetInput getInputAction = new GetInput(new WebPageRequest("useinputpage")); getInputAction.setPrompt(prompt); getInputAction.setSpeechInputMode(SpeechInputMode.AUTO); getInputAction.setDigitInputOptions(digitOptions); getInputAction.setOnInvalidInputMessages(onInvalidInputMessages); actions.add(getInputAction);
Process the response:
GetInputResult inputResult = (GetInputResult)ourRequest.getInstanceInfo().getActionResult(); if (inputResult.getInputType().equals("speech")) { List<Phrase> phrase_list = inputResult.getSpeechInput(); List<Speech> speech_list = phrase_list.get(0).getAlternatives(); String input = speech_list.get(0).getText(); // Your code here... }
-
Get an arbitrary length number and set custom retry messages:
List<TelephonyAction> actions = new ArrayList<TelephonyAction>(); Play prompt = Play.sayText("Please say your membership number."); List<Play> onInputTimeoutMessages = new ArrayList<Play>(); onInputTimeoutMessages.add(Play.playFile("silencemessage1.wav")); onInputTimeoutMessages.add(Play.playFile("silencemessage2.wav")); GetInput getInputAction = new GetInput(new WebPageRequest("useinputpage")); getInputAction.setPrompt(prompt); getInputAction.setDigitInputEnabled(false); getInputAction.setOnInputTimeoutMessages(onInputTimeoutMessages); actions.add(getInputAction);
Process the response:
GetInputResult inputResult = (GetInputResult)ourRequest.getInstanceInfo().getActionResult(); if (inputResult.getInputType().equals("speech")) { List<Phrase> phrase_list = inputResult.getSpeechInput(); List<Speech> speech_list = phrase_list.get(0).getAlternatives(); String input = speech_list.get(0).getText(); // Your code here... }
-
-
class GetInput
Represents a get input action.
Class synopsis:
# GetInput object: GetInput(next_page) # Instance methods: GetInput.set_prompt(play_action) GetInput.set_speech_input_mode(speech_input_mode) GetInput.set_speech_recognition_options(speech_recognition_options) GetInput.set_digit_input_enabled(digit_input_enabled) GetInput.set_digit_input_options(digit_input_options) GetInput.set_seconds_timeout(timeout) GetInput.set_milliseconds_inter_speech_timeout(timeout) GetInput.set_milliseconds_inter_digit_timeout(timeout) GetInput.set_milliseconds_initial_timeout(timeout) GetInput.set_on_input_timeout_messages(list_of_play_actions) GetInput.set_on_invalid_input_messages(list_of_play_actions) GetInput.set_help_digit(help_digit) GetInput.append_on_input_timeout_message(play_action=None) GetInput.append_on_invalid_input_message(play_action=None)
Get Input Result
The Record Result is represented by a dictionary. It is found within the
action result
for thenext page
.Obtaining the Get Input Result dictionary:
my_request = TelephonyRequest(request) action_result = my_request.get_action_result() if action_result.get("action") == "get_input": get_input_result = action_result.get("result")
Examples:
Get a single utterance of speech:
# Create a list of actions that will be passed to the TelephonyResponse constructor list_of_actions = [] my_input = GetInput(WebPage(url='useinputpage')) my_input.set_prompt(Play(text_to_say='Please say the reason for your call.')) my_input.set_digit_input_enabled(False) list_of_actions.append(my_input)
Process the response:
my_request = TelephonyRequest(request) action_result = my_request.get_action_result() if action_result.get("action") == "get_input": get_input_result = action_result.get("result") if get_input_result.get("input_type") == "speech": phrase_list = get_input_result.get("speech_input") speech_list = phrase_list[0].get("alternatives") input = speech_list[0].get("text").lower() if (input.find("close") != -1) and (input.find("account") != -1): print("Caller wants to close an account") # Your code here...
Get a name, specifying some likely hints, allowing speech barge in while the prompt is playing, but disabling digit input and barge in:
# Create a list of actions that will be passed to the TelephonyResponse constructor list_of_actions = [] play_action = Play(text_to_say='Please say the name of a 17th century author.') play_action.set_barge_in_on_speech(True) play_action.set_barge_in_on_digit(False) speech_recognition_opts = SpeechRecognitionOptions() word_hints = [] word_hints.append(WordHint(["Alexander Pope", "Cyrano de Bergerac", "Daniel Defoe", "John Milton", "Jonathan Swift", "Voltaire"])) speech_recognition_opts.set_word_hints(word_hints) my_input = GetInput(WebPage(url='useinputpage')) my_input.set_prompt(play_action) my_input.set_digit_input_enabled(False) my_input.set_speech_recognition_options(speech_recognition_opts) list_of_actions.append(my_input)
Process the response:
my_request = TelephonyRequest(request) action_result = my_request.get_action_result() if action_result.get("action") == "get_input": get_input_result = action_result.get("result") if get_input_result.get("input_type") == "speech": phrase_list = get_input_result.get("speech_input") speech_list = phrase_list[0].get("alternatives") input = speech_list[0].get("text").lower() if input == "jonathan swift": print("Caller said 'Jonathan Swift'") # Your code here...
Get the name of a month or the month number as entered digits, setting the inter digit timeout:
# Create a list of actions that will be passed to the TelephonyResponse constructor list_of_actions = [] my_input = GetInput(WebPage(url='useinputpage')) my_input.set_prompt(Play(text_to_say='Please say a month name or enter the month number.')) my_input.set_milliseconds_inter_digit_timeout(1500) list_of_actions.append(my_input)
Process the response:
my_request = TelephonyRequest(request) action_result = my_request.get_action_result() if action_result.get("action") == "get_input": get_input_result = action_result.get("result") if get_input_result.get("input_type") == "speech": phrase_list = get_input_result.get("speech_input") speech_list = phrase_list[0].get("alternatives") input = speech_list[0].get("text").lower() if input == "march": print("Caller said 'March'") # Your code here... elif get_input_result.get("input_type") == "digits": if get_input_result.get("digits_input") == "3": print("Caller pressed digit 3 on their keypad") # Your code here...
Get a single digit number either spoken or as an entered digit:
# Create a list of actions that will be passed to the TelephonyResponse constructor list_of_actions = [] digit_input_opts = DigitInputOptions() digit_input_opts.set_digit_count(1) my_input = GetInput(WebPage(url='useinputpage')) my_input.set_prompt(Play(text_to_say='Please say a single number from zero to nine, or press a single digit.')) my_input.set_digit_input_options(digit_input_opts) list_of_actions.append(my_input)
Process the response:
my_request = TelephonyRequest(request) action_result = my_request.get_action_result() if action_result.get("action") == "get_input": get_input_result = action_result.get("result") if get_input_result.get("input_type") == "speech": phrase_list = get_input_result.get("speech_input") speech_list = phrase_list[0].get("alternatives") input = speech_list[0].get("text").lower() if input == "7": print("Caller said '7'") # Your code here... elif get_input_result.get("input_type") == "digits": if get_input_result.get("digits_input") == "7": print("Caller pressed digit 7 on their keypad") # Your code here...
Get an eight digit number either spoken or as entered digits, specifying longer timeouts for speech input:
# Create a list of actions that will be passed to the TelephonyResponse constructor list_of_actions = [] digit_input_opts = DigitInputOptions() digit_input_opts.set_digit_count(8) my_input = GetInput(WebPage(url='useinputpage')) my_input.set_prompt(Play(text_to_say='Please say the 8 digit account number or enter it on your keypad.')) my_input.set_digit_input_options(digit_input_opts) my_input.set_milliseconds_inter_speech_timeout(2000) my_input.set_milliseconds_initial_timeout(10000) list_of_actions.append(my_input)
Process the response:
my_request = TelephonyRequest(request) action_result = my_request.get_action_result() if action_result.get("action") == "get_input": get_input_result = action_result.get("result") if get_input_result.get("input_type") == "speech": phrase_list = get_input_result.get("speech_input") speech_list = phrase_list[0].get("alternatives") input = speech_list[0].get("text").lower() # Your code here... elif get_input_result.get("input_type") == "digits": input = get_input_result.get("digits_input") # Your code here...
Get a three digit number only consisting of the digits 1-5:
# Create a list of actions that will be passed to the TelephonyResponse constructor list_of_actions = [] digit_input_opts = DigitInputOptions() digit_input_opts.set_digit_count(3) digit_input_opts.set_valid_digits("12345") invalid_messages = [] invalid_messages.append(Play(text_to_say='Please enter only digits from one to five.')) play_action = Play(text_to_say='No really!') play_action.append_text(text_to_say='Please enter only digits from one to five.') invalid_messages.append(play_action) my_input = GetInput(WebPage(url='useinputpage')) my_input.set_prompt(Play(text_to_say='Please say three digits from one to five.')) my_input.set_speech_input_mode("auto") my_input.set_digit_input_options(digit_input_opts) my_input.set_on_invalid_input_messages(invalid_messages) list_of_actions.append(my_input)
Process the response:
my_request = TelephonyRequest(request) action_result = my_request.get_action_result() if action_result.get("action") == "get_input": get_input_result = action_result.get("result") if get_input_result.get("input_type") == "speech": phrase_list = get_input_result.get("speech_input") speech_list = phrase_list[0].get("alternatives") input = speech_list[0].get("text").lower() # Your code here...
Get an arbitrary length number and set custom retry messages:
# Create a list of actions that will be passed to the TelephonyResponse constructor list_of_actions = [] timeout_messages = [] timeout_messages.append(Play(file_to_play='silencemessage1.wav')) timeout_messages.append(Play(file_to_play='silencemessage2.wav')) my_input = GetInput(WebPage(url='useinputpage')) my_input.set_prompt(Play(text_to_say='Please say your membership number.')) my_input.set_digit_input_enabled(False) my_input.set_on_input_timeout_messages(timeout_messages) list_of_actions.append(my_input)
Process the response:
my_request = TelephonyRequest(request) action_result = my_request.get_action_result() if action_result.get("action") == "get_input": get_input_result = action_result.get("result") if get_input_result.get("input_type") == "speech": phrase_list = get_input_result.get("speech_input") speech_list = phrase_list[0].get("alternatives") input = speech_list[0].get("text").lower() # Your code here...
-
The GetInput class
Introduction
Represents a get input action.
Class synopsis
class GetInput extends GetInputBase { /* methods */ public __construct(string|WebPageRequest $next_page, array $opts = null) public self setSpeechInputMode(string $mode) public self setSpeechRecognitionOptions(SpeechRecognitionOptions $opts) public self setDigitInputEnabled(boolean $enabled) public self setDigitInputOptions(DigitInputOptions $opts) public self setMillisecondsInterSpeechTimeout(int $msecs) public self setMillisecondsInterDigitTimeout(int $msecs) public self setMillisecondsInitialTimeout(int $msecs) /* inherited methods */ public self setPrompt(Play $prompt) public self setHelpDigit(string $digit) public self setOnInputTimeoutMessages(MessageList $messages) public self setOnInvalidInputMessages(MessageList $messages) public self setSecondsTimeout(int $secs) }
The GetInputResult class
Introduction
Represents the result of a get input action.
Class synopsis
class GetInputResult extends ActionResult { /* methods */ public array[Phrase] getSpeechInput() public string getDigitsInput() public string getInputType() /* inherited methods */ public string getAction() public boolean getInterrupted() }
Examples:
Get a single utterance of speech:
$prompt = \Aculab\TelephonyRestAPI\Play::sayText("Please say the reason for your call."); $getInput = new \Aculab\TelephonyRestAPI\GetInput('useinputpage'); $getInput->setPrompt($prompt) ->setDigitInputEnabled(false); $response->addAction($getInput);
Extract the spoken phrase from the action result
$info = \Aculab\TelephonyRestAPI\InstanceInfo::getInstanceInfo(); $getInputResult = $info->getActionResult(); $phrases = $getInputResult->getSpeechInput(); foreach($phrases as $phrase) { $alternatives = $phrase->getAlternatives(); foreach($alternatives as $speech) { if ($speech) { $text = $speech->getText(); $confidence = $speech->getConfidence(); /* process spoken phrase */ } } }
Get a name, specifying some likely hints, allowing speech barge in while the prompt is playing, but disabling digit input and barge in:
$prompt = \Aculab\TelephonyRestAPI\Play::sayText("Please say the name of a 17th century author."); $prompt->setBargeInOnSpeech(true) ->setBargeInOnDigit(false); $getInput = new \Aculab\TelephonyRestAPI\GetInput('useinputpage'); $getInput->setPrompt($prompt) ->setDigitInputEnabled(false); $speechRecognitionOptions = new \Aculab\TelephonyRestAPI\SpeechRecognitionOptions(); $speechRecognitionOptions->setWordHints(new \Aculab\TelephonyRestAPI\WordHint([ "Alexander Pope", "Cyrano de Bergerac", "Daniel Defoe", "John Milton", "Jonathan Swift", "Voltaire" ])); $getInput->setSpeechRecognitionOptions($speechRecognitionOptions); $response->addAction($getInput);
Extract the spoken phrase from the action result
$info = \Aculab\TelephonyRestAPI\InstanceInfo::getInstanceInfo(); $getInputResult = $info->getActionResult(); $phrases = $getInputResult->getSpeechInput(); foreach($phrases as $phrase) { $alternatives = $phrase->getAlternatives(); foreach($alternatives as $speech) { if ($speech) { $text = $speech->getText(); $confidence = $speech->getConfidence(); /* process spoken phrase */ } } }
Get the name of a month or the month number as entered digits, setting the inter digit timeout:
$prompt = \Aculab\TelephonyRestAPI\Play::sayText("Please say a month name or enter the month number."); $getInput = new \Aculab\TelephonyRestAPI\GetInput('useinputpage'); $getInput->setPrompt($prompt) ->setMillisecondsInterDigitTimeout(1500); $response->addAction($getInput);
Extract the input from the action result
$info = \Aculab\TelephonyRestAPI\InstanceInfo::getInstanceInfo(); $getInputResult = $info->getActionResult(); if ($getInputResult->getInputType() == "speech") { $phrases = $getInputResult->getSpeechInput(); foreach($phrases as $phrase) { $alternatives = $phrase->getAlternatives(); foreach($alternatives as $speech) { if ($speech) { $text = $speech->getText(); $confidence = $speech->getConfidence(); /* process spoken phrase */ } } } } else { /* digits */ $digits = $getInputResult->getDigitsInput(); /* process digits entered */ }
Get a single digit number either spoken or as an entered digit:
$prompt = \Aculab\TelephonyRestAPI\Play::sayText("Please say a single number from zero to nine, or press a single digit."); $getInput = new \Aculab\TelephonyRestAPI\GetInput('useinputpage'); $getInput->setPrompt($prompt); $digitInputOptions = new \Aculab\TelephonyRestAPI\DigitInputOptions(); $digitInputOptions->setDigitCount(1); $getInput->setDigitInputOptions($digitInputOptions); $response->addAction($getInput);
Extract the input from the action result
$info = \Aculab\TelephonyRestAPI\InstanceInfo::getInstanceInfo(); $getInputResult = $info->getActionResult(); if ($getInputResult->getInputType() == "speech") { $phrases = $getInputResult->getSpeechInput(); foreach($phrases as $phrase) { $alternatives = $phrase->getAlternatives(); foreach($alternatives as $speech) { if ($speech) { $text = $speech->getText(); $confidence = $speech->getConfidence(); /* process spoken phrase */ } } } } else { /* digits */ $digits = $getInputResult->getDigitsInput(); /* process digits entered */ }
Get an eight digit number either spoken or as entered digits, specifying longer timeouts for speech input:
$prompt = \Aculab\TelephonyRestAPI\Play::sayText("Please say the 8 digit account number or enter it on your keypad."); $getInput = new \Aculab\TelephonyRestAPI\GetInput('useinputpage'); $getInput->setPrompt($prompt) ->setMillisecondsInitialTimeout(10000) ->setMillisecondsInterSpeechTimeout(2000); $digitInputOptions = new \Aculab\TelephonyRestAPI\DigitInputOptions(); $digitInputOptions->setDigitCount(8); $getInput->setDigitInputOptions($digitInputOptions); $response->addAction($getInput);
Extract the input from the action result
$info = \Aculab\TelephonyRestAPI\InstanceInfo::getInstanceInfo(); $getInputResult = $info->getActionResult(); if ($getInputResult->getInputType() == "speech") { $phrases = $getInputResult->getSpeechInput(); foreach($phrases as $phrase) { $alternatives = $phrase->getAlternatives(); foreach($alternatives as $speech) { if ($speech) { $text = $speech->getText(); $confidence = $speech->getConfidence(); /* process spoken phrase */ } } } } else { /* digits */ $digits = $getInputResult->getDigitsInput(); /* process digits entered */ }
Get a three digit number only consisting of the digits 1-5:
$prompt = \Aculab\TelephonyRestAPI\Play::sayText("Please say three digits from one to five."); $getInput = new \Aculab\TelephonyRestAPI\GetInput('useinputpage'); $getInput->setPrompt($prompt) ->setSpeechInputMode("auto"); $digitInputOptions = new \Aculab\TelephonyRestAPI\DigitInputOptions(); $digitInputOptions->setDigitCount(3) ->setValidDigits("12345"); $getInput->setDigitInputOptions($digitInputOptions); $invalidMessages = new \Aculab\TelephonyRestAPI\MessageList(); $invalidMessages->addMessage(\Aculab\TelephonyRestAPI\Play::sayText("Please enter only digits from one to five.")) ->addMessage(\Aculab\TelephonyRestAPI\Play::sayText("No really!")->addText("Please enter only digits from one to five.")); $getInput->setOnInvalidInputMessages($invalidMessages); $response->addAction($getInput);
Extract the input from the action result
$info = \Aculab\TelephonyRestAPI\InstanceInfo::getInstanceInfo(); $getInputResult = $info->getActionResult(); if ($getInputResult->getInputType() == "speech") { $phrases = $getInputResult->getSpeechInput(); foreach($phrases as $phrase) { $alternatives = $phrase->getAlternatives(); foreach($alternatives as $speech) { if ($speech) { $text = $speech->getText(); $confidence = $speech->getConfidence(); /* process spoken phrase */ } } } } else { /* digits */ $digits = $getInputResult->getDigitsInput(); /* process digits entered */ }
Get an arbitrary length number and set custom retry messages:
$prompt = \Aculab\TelephonyRestAPI\Play::sayText("Please say your membership number."); $getInput = new \Aculab\TelephonyRestAPI\GetInput('useinputpage'); $getInput->setPrompt($prompt) ->setDigitInputEnabled(false); $timeoutMessages = new \Aculab\TelephonyRestAPI\MessageList(); $timeoutMessages->addMessage(\Aculab\TelephonyRestAPI\Play::playFile("silencemessage1.wav")) ->addMessage(\Aculab\TelephonyRestAPI\Play::playFile("silencemessage2.wav")); $getInput->setOnInputTimeoutMessages($timeoutMessages); $response->addAction($getInput);
Extract the spoken phrase from the action result
$info = \Aculab\TelephonyRestAPI\InstanceInfo::getInstanceInfo(); $getInputResult = $info->getActionResult(); $phrases = $getInputResult->getSpeechInput(); foreach($phrases as $phrase) { $alternatives = $phrase->getAlternatives(); foreach($alternatives as $speech) { if ($speech) { $text = $speech->getText(); $confidence = $speech->getConfidence(); /* process spoken phrase */ } } }